mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-20 09:31:31 +00:00
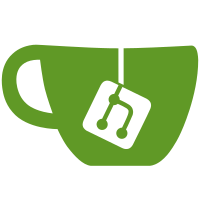
* Split out BehaviorMessage class changes from PR #1452 * remove <string_view> inclusion in ActionContext.h * add the arguments nullptr check back in * remove redundant std::string constructor calls * Update AddStripMessage.cpp - change push_back to emplace_back
35 lines
1.1 KiB
C++
35 lines
1.1 KiB
C++
#include "Action.h"
|
|
#include "Amf3.h"
|
|
|
|
Action::Action(const AMFArrayValue* arguments) {
|
|
for (const auto& [paramName, paramValue] : arguments->GetAssociative()) {
|
|
if (paramName == "Type") {
|
|
if (paramValue->GetValueType() != eAmf::String) continue;
|
|
m_Type = static_cast<AMFStringValue*>(paramValue)->GetValue();
|
|
} else {
|
|
m_ValueParameterName = paramName;
|
|
// Message is the only known string parameter
|
|
if (m_ValueParameterName == "Message") {
|
|
if (paramValue->GetValueType() != eAmf::String) continue;
|
|
m_ValueParameterString = static_cast<AMFStringValue*>(paramValue)->GetValue();
|
|
} else {
|
|
if (paramValue->GetValueType() != eAmf::Double) continue;
|
|
m_ValueParameterDouble = static_cast<AMFDoubleValue*>(paramValue)->GetValue();
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
void Action::SendBehaviorBlocksToClient(AMFArrayValue& args) const {
|
|
auto* const actionArgs = args.PushArray();
|
|
actionArgs->Insert("Type", m_Type);
|
|
|
|
if (m_ValueParameterName.empty()) return;
|
|
|
|
if (m_ValueParameterName == "Message") {
|
|
actionArgs->Insert(m_ValueParameterName, m_ValueParameterString);
|
|
} else {
|
|
actionArgs->Insert(m_ValueParameterName, m_ValueParameterDouble);
|
|
}
|
|
}
|