mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-11-10 10:18:21 +00:00
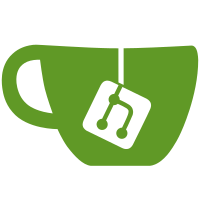
* Move CDClientManager to be a namespace Tested that worlds still load data as expected. Had no use being a singleton anyways. * Move cdclient data storage to tu local containers Allows some data from these containers to be saved on object by reference instead of always needing to copy. iteration 2 - move all unnamed namespace containers to a singular spot - use macro for template specialization and variable declaration - use templates to allow for as little copy paste of types and functions as possible * remember to use typename! compiler believes T::StorageType is accessing a member, not a type. * Update CDClientManager.cpp * move to cpp?
51 lines
1.3 KiB
C++
51 lines
1.3 KiB
C++
#pragma once
|
|
|
|
#include "CDClientDatabase.h"
|
|
#include "CDClientManager.h"
|
|
#include "Singleton.h"
|
|
#include "DluAssert.h"
|
|
|
|
#include <functional>
|
|
#include <string>
|
|
#include <vector>
|
|
#include <map>
|
|
#include <cstdint>
|
|
|
|
// CPPLinq
|
|
#ifdef _WIN32
|
|
#define NOMINMAX
|
|
// windows.h has min and max macros that breaks cpplinq
|
|
#endif
|
|
#include "cpplinq.hpp"
|
|
|
|
// Used for legacy
|
|
#define UNUSED(x)
|
|
|
|
// Enable this to skip some unused columns in some tables
|
|
#define UNUSED_COLUMN(v)
|
|
|
|
// Use this to skip unused defaults for unused entries in some tables
|
|
#define UNUSED_ENTRY(v, x)
|
|
|
|
#pragma warning (disable : 4244) //Disable double to float conversion warnings
|
|
// #pragma warning (disable : 4715) //Disable "not all control paths return a value"
|
|
|
|
template<class Table, typename Storage>
|
|
class CDTable : public Singleton<Table> {
|
|
public:
|
|
typedef Storage StorageType;
|
|
|
|
protected:
|
|
virtual ~CDTable() = default;
|
|
|
|
// If you need these for a specific table, override it such that there is a public variant.
|
|
[[nodiscard]] StorageType& GetEntriesMutable() const {
|
|
return CDClientManager::GetEntriesMutable<Table>();
|
|
}
|
|
|
|
// If you need these for a specific table, override it such that there is a public variant.
|
|
[[nodiscard]] const StorageType& GetEntries() const {
|
|
return GetEntriesMutable();
|
|
}
|
|
};
|