mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-19 17:11:32 +00:00
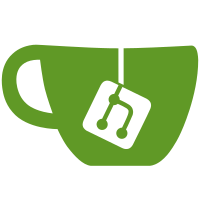
* Move CDClientManager to be a namespace Tested that worlds still load data as expected. Had no use being a singleton anyways. * Move cdclient data storage to tu local containers Allows some data from these containers to be saved on object by reference instead of always needing to copy. iteration 2 - move all unnamed namespace containers to a singular spot - use macro for template specialization and variable declaration - use templates to allow for as little copy paste of types and functions as possible * remember to use typename! compiler believes T::StorageType is accessing a member, not a type. * Update CDClientManager.cpp * move to cpp?
41 lines
1.0 KiB
C++
41 lines
1.0 KiB
C++
#include "CDBrickIDTableTable.h"
|
|
|
|
void CDBrickIDTableTable::LoadValuesFromDatabase() {
|
|
|
|
// First, get the size of the table
|
|
uint32_t size = 0;
|
|
auto tableSize = CDClientDatabase::ExecuteQuery("SELECT COUNT(*) FROM BrickIDTable");
|
|
while (!tableSize.eof()) {
|
|
size = tableSize.getIntField(0, 0);
|
|
|
|
tableSize.nextRow();
|
|
}
|
|
|
|
tableSize.finalize();
|
|
|
|
// Reserve the size
|
|
auto& entries = GetEntriesMutable();
|
|
entries.reserve(size);
|
|
|
|
// Now get the data
|
|
auto tableData = CDClientDatabase::ExecuteQuery("SELECT * FROM BrickIDTable");
|
|
while (!tableData.eof()) {
|
|
CDBrickIDTable entry;
|
|
entry.NDObjectID = tableData.getIntField("NDObjectID", -1);
|
|
entry.LEGOBrickID = tableData.getIntField("LEGOBrickID", -1);
|
|
|
|
entries.push_back(entry);
|
|
tableData.nextRow();
|
|
}
|
|
|
|
tableData.finalize();
|
|
}
|
|
|
|
std::vector<CDBrickIDTable> CDBrickIDTableTable::Query(std::function<bool(CDBrickIDTable)> predicate) {
|
|
std::vector<CDBrickIDTable> data = cpplinq::from(GetEntries())
|
|
>> cpplinq::where(predicate)
|
|
>> cpplinq::to_vector();
|
|
|
|
return data;
|
|
}
|