mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-19 09:01:31 +00:00
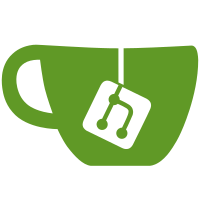
* First iteration of pack reader and interface * Fix memory leak and remove logs * Complete packed asset interface and begin on file loading replacement * Implement proper BinaryIO error * Improve AssetMemoryBuffer for reading and implement more reading * Repair more file loading code and improve how navmeshes are loaded * Missing checks implementation * Revert addition of Manifest class and migration changes * Resolved all feedback.
41 lines
852 B
C++
41 lines
852 B
C++
#pragma once
|
|
|
|
#include <cstdint>
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
#include <filesystem>
|
|
|
|
#include "Pack.h"
|
|
|
|
#pragma pack(push, 1)
|
|
struct PackFileIndex {
|
|
uint32_t m_Crc;
|
|
int32_t m_LowerCrc;
|
|
int32_t m_UpperCrc;
|
|
uint32_t m_PackFileIndex;
|
|
uint32_t m_IsCompressed; // u32 bool?
|
|
};
|
|
#pragma pack(pop)
|
|
|
|
class PackIndex {
|
|
public:
|
|
PackIndex(const std::filesystem::path& filePath);
|
|
~PackIndex();
|
|
|
|
const std::vector<std::string>& GetPackPaths() { return m_PackPaths; }
|
|
const std::vector<PackFileIndex>& GetPackFileIndices() { return m_PackFileIndices; }
|
|
const std::vector<Pack*>& GetPacks() { return m_Packs; }
|
|
private:
|
|
std::ifstream m_FileStream;
|
|
|
|
uint32_t m_Version;
|
|
|
|
uint32_t m_PackPathCount;
|
|
std::vector<std::string> m_PackPaths;
|
|
uint32_t m_PackFileIndexCount;
|
|
std::vector<PackFileIndex> m_PackFileIndices;
|
|
|
|
std::vector<Pack*> m_Packs;
|
|
};
|