mirror of
https://github.com/python-kasa/python-kasa.git
synced 2025-05-16 19:41:09 +00:00
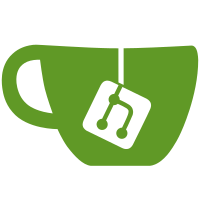
* Add support for new-style protocol Newer devices (including my LB130) seem to include the request length in the previously empty message header, and ignore requests that lack it. They also don't send an empty packet as the final part of a response, which can lead to hangs. Add support for this, with luck not breaking existing devices in the process. * Fix tests We now include the request length in the encrypted packet header, so strip the header rather than assuming that it's just zeroes. * Create a SmartDevice parent class Add a generic SmartDevice class that SmartPlug can inherit from, in preparation for adding support for other device types. * Add support for TP-Link smartbulbs These bulbs use the same protocol as the smart plugs, but have additional commands for controlling bulb-specific features. In addition, the bulbs have their emeter under a different target and return responses that include the energy unit in the key names. * Add tests for bulbs Not entirely comprehensive, but has pretty much the same level of testing as plugs
Refactor & add unittests for almost all functionality, add tox for running tests on py27 and py35 (#17)
Refactor & add unittests for almost all functionality, add tox for running tests on py27 and py35 (#17)
pyHS100
Python Library to control TPLink Switch (HS100 / HS110)
Usage
For all available API functions run help(SmartPlug)
from pyHS100 import SmartPlug
from pprint import pformat as pf
plug = SmartPlug("192.168.250.186")
print("Alias, type and supported features: %s" % (plug.identify(),))
print("Hardware: %s" % pf(plug.hw_info))
print("Full sysinfo: %s" % pf(plug.get_sysinfo())) # this prints lots of information about the device
Time information
print("Current time: %s" % plug.time)
print("Timezone: %s" % plug.timezone)
Getting and setting the name
print("Alias: %s" % plug.alias)
plug.alias = "My New Smartplug"
State & switching
print("Current state: %s" % plug.state)
plug.turn_off()
plug.turn_on()
or
plug.state = "ON"
plug.state = "OFF"
Getting emeter status (on HS110)
print("Current consumption: %s" % plug.get_emeter_realtime())
print("Per day: %s" % plug.get_emeter_daily(year=2016, month=12))
print("Per month: %s" % plug.get_emeter_monthly(year=2016))
Switching the led
print("Current LED state: %s" % plug.led)
plug.led = False # turn off led
print("New LED state: %s" % plug.led)
Example
There is also a simple tool for testing connectivity in examples, to use:
python -m examples.cli <ip>
Discovering devices
python3 -m examples.discover
Languages
Python
100%