mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-19 17:11:32 +00:00
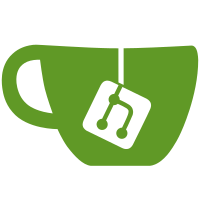
* Logger: Rename logger to Logger from dLogger * Logger: Add compile time filename Fix include issues Add writers Add macros Add macro to force compilation * Logger: Replace calls with macros Allows for filename and line number to be logged * Logger: Add comments and remove extra define Logger: Replace with unique_ptr also flush console at exit. regular file writer should be flushed on file close. Logger: Remove constexpr on variable * Logger: Simplify code * Update Logger.cpp
57 lines
1.9 KiB
C++
57 lines
1.9 KiB
C++
#include "PropertyVendorComponent.h"
|
|
|
|
#include "PropertyDataMessage.h"
|
|
#include "GameMessages.h"
|
|
#include "Character.h"
|
|
#include "EntityManager.h"
|
|
#include "dZoneManager.h"
|
|
#include "Game.h"
|
|
#include "Logger.h"
|
|
#include "PropertyManagementComponent.h"
|
|
#include "UserManager.h"
|
|
|
|
PropertyVendorComponent::PropertyVendorComponent(Entity* parent) : Component(parent) {
|
|
}
|
|
|
|
void PropertyVendorComponent::OnUse(Entity* originator) {
|
|
if (PropertyManagementComponent::Instance() == nullptr) return;
|
|
|
|
OnQueryPropertyData(originator, originator->GetSystemAddress());
|
|
|
|
if (PropertyManagementComponent::Instance()->GetOwnerId() == LWOOBJID_EMPTY) {
|
|
LOG("Property vendor opening!");
|
|
|
|
GameMessages::SendOpenPropertyVendor(m_Parent->GetObjectID(), originator->GetSystemAddress());
|
|
|
|
return;
|
|
}
|
|
}
|
|
|
|
void PropertyVendorComponent::OnQueryPropertyData(Entity* originator, const SystemAddress& sysAddr) {
|
|
if (PropertyManagementComponent::Instance() == nullptr) return;
|
|
|
|
PropertyManagementComponent::Instance()->OnQueryPropertyData(originator, sysAddr, m_Parent->GetObjectID());
|
|
}
|
|
|
|
void PropertyVendorComponent::OnBuyFromVendor(Entity* originator, const bool confirmed, const LOT lot, const uint32_t count) {
|
|
if (PropertyManagementComponent::Instance() == nullptr) return;
|
|
|
|
if (PropertyManagementComponent::Instance()->Claim(originator->GetObjectID()) == false) {
|
|
LOG("FAILED TO CLAIM PROPERTY. PLAYER ID IS %llu", originator->GetObjectID());
|
|
return;
|
|
}
|
|
|
|
GameMessages::SendPropertyRentalResponse(m_Parent->GetObjectID(), 0, 0, 0, 0, originator->GetSystemAddress());
|
|
|
|
auto* controller = Game::zoneManager->GetZoneControlObject();
|
|
|
|
controller->OnFireEventServerSide(m_Parent, "propertyRented");
|
|
|
|
PropertyManagementComponent::Instance()->SetOwner(originator);
|
|
|
|
PropertyManagementComponent::Instance()->OnQueryPropertyData(originator, originator->GetSystemAddress());
|
|
|
|
LOG("Fired event; (%d) (%i) (%i)", confirmed, lot, count);
|
|
}
|
|
|