mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2025-07-07 04:00:02 +00:00
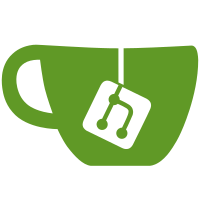
* EntityManager: ranges and cleanup Use LWOOBJID for ghosting entities use ranges::views::values for associative container iteration remove dead code comment magic numbers little bit of optimization (not enough to be game changing or take the time to measure, they are free speedups anyways, we take those) use cstdint types * use size_t * use lwoobjid for ghost candidate
55 lines
1.4 KiB
C++
55 lines
1.4 KiB
C++
#ifndef __GHOSTCOMPONENT__H__
|
|
#define __GHOSTCOMPONENT__H__
|
|
|
|
#include "Component.h"
|
|
#include "eReplicaComponentType.h"
|
|
#include <unordered_set>
|
|
|
|
class NiPoint3;
|
|
|
|
class GhostComponent final : public Component {
|
|
public:
|
|
static inline const eReplicaComponentType ComponentType = eReplicaComponentType::GHOST;
|
|
GhostComponent(Entity* parent);
|
|
~GhostComponent() override;
|
|
|
|
void SetGhostOverride(bool value) { m_GhostOverride = value; };
|
|
|
|
const NiPoint3& GetGhostReferencePoint() const { return m_GhostOverride ? m_GhostOverridePoint : m_GhostReferencePoint; };
|
|
|
|
const NiPoint3& GetOriginGhostReferencePoint() const { return m_GhostReferencePoint; };
|
|
|
|
const NiPoint3& GetGhostOverridePoint() const { return m_GhostOverridePoint; };
|
|
|
|
bool GetGhostOverride() const { return m_GhostOverride; };
|
|
|
|
void SetGhostReferencePoint(const NiPoint3& value);
|
|
|
|
void SetGhostOverridePoint(const NiPoint3& value);
|
|
|
|
void AddLimboConstruction(const LWOOBJID objectId);
|
|
|
|
void RemoveLimboConstruction(const LWOOBJID objectId);
|
|
|
|
void ConstructLimboEntities();
|
|
|
|
void ObserveEntity(const LWOOBJID id);
|
|
|
|
bool IsObserved(const LWOOBJID id);
|
|
|
|
void GhostEntity(const LWOOBJID id);
|
|
|
|
private:
|
|
NiPoint3 m_GhostReferencePoint;
|
|
|
|
NiPoint3 m_GhostOverridePoint;
|
|
|
|
std::unordered_set<LWOOBJID> m_ObservedEntities;
|
|
|
|
std::unordered_set<LWOOBJID> m_LimboConstructions;
|
|
|
|
bool m_GhostOverride;
|
|
};
|
|
|
|
#endif //!__GHOSTCOMPONENT__H__
|