mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-20 01:21:32 +00:00
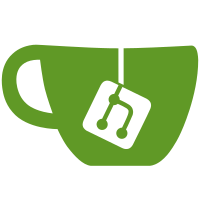
* allow usage of NiPoint3 and NiQuaternion in constexpr context * removed .cpp files entirely * moving circular dependency circumvention stuff to an .inl file * real world usage!!!!! * reverting weird branch cross-pollination * removing more weird branch cross-pollination * remove comment * added inverse header guard to inl file * Update NiPoint3.inl * trying different constructor syntax * reorganize into .inl files for readability * uncomment include * moved non-constexpr definitions to cpp file * moved static definitions back to inl files * testing fix * moved constants into seperate namespace * Undo change in build-and-test.yml * nodiscard
58 lines
1.4 KiB
C++
58 lines
1.4 KiB
C++
#include "GhostComponent.h"
|
|
|
|
GhostComponent::GhostComponent(Entity* parent) : Component(parent) {
|
|
m_GhostReferencePoint = NiPoint3Constant::ZERO;
|
|
m_GhostOverridePoint = NiPoint3Constant::ZERO;
|
|
m_GhostOverride = false;
|
|
}
|
|
|
|
GhostComponent::~GhostComponent() {
|
|
for (auto& observedEntity : m_ObservedEntities) {
|
|
if (observedEntity == 0) continue;
|
|
|
|
auto* entity = Game::entityManager->GetGhostCandidate(observedEntity);
|
|
if (!entity) continue;
|
|
|
|
entity->SetObservers(entity->GetObservers() - 1);
|
|
}
|
|
}
|
|
|
|
void GhostComponent::SetGhostReferencePoint(const NiPoint3& value) {
|
|
m_GhostReferencePoint = value;
|
|
}
|
|
|
|
void GhostComponent::SetGhostOverridePoint(const NiPoint3& value) {
|
|
m_GhostOverridePoint = value;
|
|
}
|
|
|
|
void GhostComponent::AddLimboConstruction(LWOOBJID objectId) {
|
|
m_LimboConstructions.insert(objectId);
|
|
}
|
|
|
|
void GhostComponent::RemoveLimboConstruction(LWOOBJID objectId) {
|
|
m_LimboConstructions.erase(objectId);
|
|
}
|
|
|
|
void GhostComponent::ConstructLimboEntities() {
|
|
for (const auto& objectId : m_LimboConstructions) {
|
|
auto* entity = Game::entityManager->GetEntity(objectId);
|
|
if (!entity) continue;
|
|
|
|
Game::entityManager->ConstructEntity(entity, m_Parent->GetSystemAddress());
|
|
}
|
|
|
|
m_LimboConstructions.clear();
|
|
}
|
|
|
|
void GhostComponent::ObserveEntity(int32_t id) {
|
|
m_ObservedEntities.insert(id);
|
|
}
|
|
|
|
bool GhostComponent::IsObserved(int32_t id) {
|
|
return m_ObservedEntities.contains(id);
|
|
}
|
|
|
|
void GhostComponent::GhostEntity(int32_t id) {
|
|
m_ObservedEntities.erase(id);
|
|
}
|