mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-11-12 19:28:21 +00:00
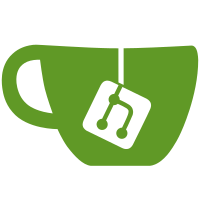
Fixes #1222 addresses an issue where the death behavior of a destructible component was not being respected and enemies with destroyable components that had special death animations were not able to play the animation on death. This pr adds in the hardcoded constant the client uses for the same metric of 12 seconds. Tested that claiming Nimbus Rock and completing the property guards mission allows him to vacuum away and then network the destruction packet 12 seconds later.
58 lines
1.6 KiB
C++
58 lines
1.6 KiB
C++
#include "AgPropguards.h"
|
|
#include "Character.h"
|
|
#include "GameMessages.h"
|
|
#include "EntityManager.h"
|
|
#include "dZoneManager.h"
|
|
#include "eMissionState.h"
|
|
|
|
void AgPropguards::OnMissionDialogueOK(Entity* self, Entity* target, int missionID, eMissionState missionState) {
|
|
auto* character = target->GetCharacter();
|
|
if (character == nullptr)
|
|
return;
|
|
|
|
const auto flag = GetFlagForMission(missionID);
|
|
if (flag == 0)
|
|
return;
|
|
|
|
if ((missionState == eMissionState::AVAILABLE || missionState == eMissionState::ACTIVE)
|
|
&& !character->GetPlayerFlag(flag)) {
|
|
// If the player just started the mission, play a cinematic highlighting the target
|
|
GameMessages::SendPlayCinematic(target->GetObjectID(), u"MissionCam", target->GetSystemAddress());
|
|
} else if (missionState == eMissionState::READY_TO_COMPLETE) {
|
|
// Makes the guard disappear once the mission has been completed
|
|
const auto zoneControlID = Game::entityManager->GetZoneControlEntity()->GetObjectID();
|
|
GameMessages::SendNotifyClientObject(zoneControlID, u"GuardChat", 0, 0, self->GetObjectID(),
|
|
"", UNASSIGNED_SYSTEM_ADDRESS);
|
|
|
|
self->AddCallbackTimer(5.0f, [self]() {
|
|
auto spawnerName = self->GetVar<std::string>(u"spawner_name");
|
|
if (spawnerName.empty())
|
|
spawnerName = "Guard";
|
|
|
|
auto spawners = Game::zoneManager->GetSpawnersByName(spawnerName);
|
|
for (auto* spawner : spawners) {
|
|
spawner->Deactivate();
|
|
}
|
|
|
|
self->Smash();
|
|
});
|
|
}
|
|
}
|
|
|
|
int32_t AgPropguards::GetFlagForMission(uint32_t missionID) {
|
|
switch (missionID) {
|
|
case 872:
|
|
return 97;
|
|
case 873:
|
|
return 98;
|
|
case 874:
|
|
return 99;
|
|
case 1293:
|
|
return 118;
|
|
case 1322:
|
|
return 122;
|
|
default:
|
|
return 0;
|
|
}
|
|
}
|