mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-11-09 17:58:20 +00:00
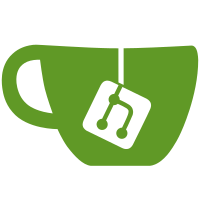
* allow usage of NiPoint3 and NiQuaternion in constexpr context * removed .cpp files entirely * moving circular dependency circumvention stuff to an .inl file * real world usage!!!!! * reverting weird branch cross-pollination * removing more weird branch cross-pollination * remove comment * added inverse header guard to inl file * Update NiPoint3.inl * trying different constructor syntax * reorganize into .inl files for readability * uncomment include * moved non-constexpr definitions to cpp file * moved static definitions back to inl files * testing fix * moved constants into seperate namespace * Undo change in build-and-test.yml * nodiscard
51 lines
1.3 KiB
C
51 lines
1.3 KiB
C
#ifndef __POSITIONUPDATE__H__
|
|
#define __POSITIONUPDATE__H__
|
|
|
|
#include "NiPoint3.h"
|
|
#include "NiQuaternion.h"
|
|
|
|
|
|
struct RemoteInputInfo {
|
|
RemoteInputInfo() {
|
|
m_RemoteInputX = 0;
|
|
m_RemoteInputY = 0;
|
|
m_IsPowersliding = false;
|
|
m_IsModified = false;
|
|
}
|
|
|
|
void operator=(const RemoteInputInfo& other) {
|
|
m_RemoteInputX = other.m_RemoteInputX;
|
|
m_RemoteInputY = other.m_RemoteInputY;
|
|
m_IsPowersliding = other.m_IsPowersliding;
|
|
m_IsModified = other.m_IsModified;
|
|
}
|
|
|
|
bool operator==(const RemoteInputInfo& other) {
|
|
return m_RemoteInputX == other.m_RemoteInputX && m_RemoteInputY == other.m_RemoteInputY && m_IsPowersliding == other.m_IsPowersliding && m_IsModified == other.m_IsModified;
|
|
}
|
|
|
|
float m_RemoteInputX;
|
|
float m_RemoteInputY;
|
|
bool m_IsPowersliding;
|
|
bool m_IsModified;
|
|
};
|
|
|
|
struct LocalSpaceInfo {
|
|
LWOOBJID objectId = LWOOBJID_EMPTY;
|
|
NiPoint3 position = NiPoint3Constant::ZERO;
|
|
NiPoint3 linearVelocity = NiPoint3Constant::ZERO;
|
|
};
|
|
|
|
struct PositionUpdate {
|
|
NiPoint3 position = NiPoint3Constant::ZERO;
|
|
NiQuaternion rotation = NiQuaternionConstant::IDENTITY;
|
|
bool onGround = false;
|
|
bool onRail = false;
|
|
NiPoint3 velocity = NiPoint3Constant::ZERO;
|
|
NiPoint3 angularVelocity = NiPoint3Constant::ZERO;
|
|
LocalSpaceInfo localSpaceInfo;
|
|
RemoteInputInfo remoteInputInfo;
|
|
};
|
|
|
|
#endif //!__POSITIONUPDATE__H__
|