mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-20 01:21:32 +00:00
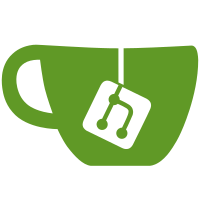
* Components: Make ComponentType inline Prevents the next commits ODR violation * Components: Add new components * Entity: Add headers inline script component ComponentType * Components: Flip constructor argument order Entity comes first always * Entity: Add generic AddComponent Allows for much easier adding of components and is error proof by not allowing the user to add more than 1 of a specific component type to an Entity. * Entity: Migrate all component constructors Move all to the new variadic templates AddComponent function to reduce clutter and ways the component map is modified. The new function makes no assumptions. Component is assumed to not exist and is checked for with operator[]. This will construct a null component which will then be newed if the component didnt exist, or it will just get the current component if it does already exist. No new component will be allocated or constructed if the component already exists and the already existing pointer is returned instead. * Entity: Add placement new For the case where the component may already exist, use a placement new to construct the component again, it would be constructed again, but would not need to go through the allocator. * Entity: Add comments on likely new code * Tests: Fix tests * Update Entity.cpp * Update SGCannon.cpp * Entity: call destructor when re-constructing * Update Entity.cpp Update Entity.cpp --------- Co-authored-by: Aaron Kimbrell <aronwk.aaron@gmail.com>
139 lines
3.3 KiB
C++
139 lines
3.3 KiB
C++
#pragma once
|
|
#include "dCommonVars.h"
|
|
#include "NiPoint3.h"
|
|
#include "Entity.h"
|
|
#include "Component.h"
|
|
#include "eReplicaComponentType.h"
|
|
|
|
/**
|
|
* Parameters for the shooting gallery that change during playtime
|
|
*/
|
|
struct DynamicShootingGalleryParams {
|
|
|
|
/**
|
|
* The distance from the camera to the barrel
|
|
*/
|
|
Vector3 cameraBarrelOffset;
|
|
|
|
/**
|
|
* The area the barrel is looking at
|
|
*/
|
|
Vector3 facing;
|
|
|
|
/**
|
|
* The velocity of the cannonballs
|
|
*/
|
|
double_t cannonVelocity;
|
|
|
|
/**
|
|
* The max firerate of the cannon
|
|
*/
|
|
double_t cannonRefireRate;
|
|
|
|
/**
|
|
* The min distance the cannonballs traverse
|
|
*/
|
|
double_t cannonMinDistance;
|
|
|
|
/**
|
|
* The angle at which the cannon is shooting
|
|
*/
|
|
float_t cannonAngle;
|
|
|
|
/**
|
|
* The timeout between cannon shots
|
|
*/
|
|
float_t cannonTimeout;
|
|
|
|
/**
|
|
* The FOV while in the canon
|
|
*/
|
|
float_t cannonFOV;
|
|
};
|
|
|
|
/**
|
|
* Parameters for the shooting gallery that don't change over time
|
|
*/
|
|
struct StaticShootingGalleryParams {
|
|
|
|
/**
|
|
* The position of the camera
|
|
*/
|
|
Vector3 cameraPosition;
|
|
|
|
/**
|
|
* The position that the camera is looking at
|
|
*/
|
|
Vector3 cameraLookatPosition;
|
|
};
|
|
|
|
/**
|
|
* A very ancient component that was used to guide shooting galleries, it's still kind of used but a lot of logic is
|
|
* also in the related scripts.
|
|
*/
|
|
class ShootingGalleryComponent : public Component {
|
|
public:
|
|
inline static const eReplicaComponentType ComponentType = eReplicaComponentType::SHOOTING_GALLERY;
|
|
|
|
explicit ShootingGalleryComponent(Entity* parent);
|
|
~ShootingGalleryComponent();
|
|
void Serialize(RakNet::BitStream* outBitStream, bool isInitialUpdate) override;
|
|
|
|
/**
|
|
* Returns the static params for the shooting gallery
|
|
* @return the static params for the shooting gallery
|
|
*/
|
|
const StaticShootingGalleryParams& GetStaticParams() const { return m_StaticParams; };
|
|
|
|
/**
|
|
* Sets the static parameters for the shooting gallery, see `StaticShootingGalleryParams`
|
|
* @param params the params to set
|
|
*/
|
|
void SetStaticParams(const StaticShootingGalleryParams& params);
|
|
|
|
/**
|
|
* Returns the dynamic params for the shooting gallery
|
|
* @return the dynamic params for the shooting gallery
|
|
*/
|
|
const DynamicShootingGalleryParams& GetDynamicParams() const { return m_DynamicParams; };
|
|
|
|
/**
|
|
* Sets the mutable params for the shooting gallery, see `DynamicShootingGalleryParams`
|
|
* @param params the params to set
|
|
*/
|
|
void SetDynamicParams(const DynamicShootingGalleryParams& params);
|
|
|
|
/**
|
|
* Sets the entity that's currently playing the shooting gallery
|
|
* @param playerID the entity to set
|
|
*/
|
|
void SetCurrentPlayerID(LWOOBJID playerID) { m_CurrentPlayerID = playerID; m_Dirty = true; };
|
|
|
|
/**
|
|
* Returns the player that's currently playing the shooting gallery
|
|
* @return the player that's currently playing the shooting gallery
|
|
*/
|
|
LWOOBJID GetCurrentPlayerID() const { return m_CurrentPlayerID; };
|
|
private:
|
|
|
|
/**
|
|
* The player that's currently playing the shooting gallery
|
|
*/
|
|
LWOOBJID m_CurrentPlayerID = LWOOBJID_EMPTY;
|
|
|
|
/**
|
|
* The static parameters for the shooting gallery, see `StaticShootingGalleryParams`
|
|
*/
|
|
StaticShootingGalleryParams m_StaticParams{};
|
|
|
|
/**
|
|
* The dynamic params for the shooting gallery, see `DynamicShootingGalleryParams`
|
|
*/
|
|
DynamicShootingGalleryParams m_DynamicParams{};
|
|
|
|
/**
|
|
* Whether or not the component should be serialized
|
|
*/
|
|
bool m_Dirty = false;
|
|
};
|