mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-11-15 12:48:20 +00:00
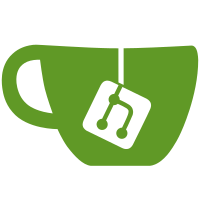
* chore: Remove dpEntity pointers from collision checking * Update fn documentation in ProximityMonitorComponent.h * use more idiomatic method to calculate vector index * feedback * missed a ranges::find replacement * adjust for feedback. last changes tonight. * okay, also remove unneeded include. then sleep. * for real tho * update to use unordered_set instead of set
43 lines
1.4 KiB
C++
43 lines
1.4 KiB
C++
#include "WanderingVendor.h"
|
|
#include "MovementAIComponent.h"
|
|
#include "ProximityMonitorComponent.h"
|
|
#include <ranges>
|
|
|
|
void WanderingVendor::OnStartup(Entity* self) {
|
|
auto movementAIComponent = self->GetComponent<MovementAIComponent>();
|
|
if (!movementAIComponent) return;
|
|
self->SetProximityRadius(10, "playermonitor");
|
|
}
|
|
|
|
void WanderingVendor::OnProximityUpdate(Entity* self, Entity* entering, std::string name, std::string status) {
|
|
if (status == "ENTER" && entering->IsPlayer()) {
|
|
auto movementAIComponent = self->GetComponent<MovementAIComponent>();
|
|
if (!movementAIComponent) return;
|
|
movementAIComponent->Pause();
|
|
self->CancelTimer("startWalking");
|
|
} else if (status == "LEAVE") {
|
|
auto* proximityMonitorComponent = self->GetComponent<ProximityMonitorComponent>();
|
|
if (!proximityMonitorComponent) self->AddComponent<ProximityMonitorComponent>();
|
|
|
|
const auto proxObjs = proximityMonitorComponent->GetProximityObjects("playermonitor");
|
|
bool foundPlayer = false;
|
|
for (const auto id : proxObjs) {
|
|
auto* entity = Game::entityManager->GetEntity(id);
|
|
if (entity && entity->IsPlayer()) {
|
|
foundPlayer = true;
|
|
break;
|
|
}
|
|
}
|
|
|
|
if (!foundPlayer) self->AddTimer("startWalking", 1.5);
|
|
}
|
|
}
|
|
|
|
void WanderingVendor::OnTimerDone(Entity* self, std::string timerName) {
|
|
if (timerName == "startWalking") {
|
|
auto movementAIComponent = self->GetComponent<MovementAIComponent>();
|
|
if (!movementAIComponent) return;
|
|
movementAIComponent->Resume();
|
|
}
|
|
}
|