mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-21 01:51:31 +00:00
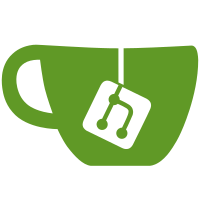
Clean up macros more tomorrow Cleanup and optimize CDActivities table Remove unused include Further work on CDActivityRewards Update MasterServer.cpp Further animations work Activities still needs work for a better PK. fix type All of these replacements worked Create internal interface for animations Allows for user to just call GetAnimationTIme or PlayAnimation rather than passing in arbitrary true false statements
85 lines
3.5 KiB
C++
85 lines
3.5 KiB
C++
#include "CDAnimationsTable.h"
|
|
#include "GeneralUtils.h"
|
|
#include "Game.h"
|
|
|
|
bool CDAnimationsTable::CacheData(CppSQLite3Statement queryToCache) {
|
|
auto tableData = queryToCache.execQuery();
|
|
// If we received a bad lookup, cache it anyways so we do not run the query again.
|
|
if (tableData.eof()) return false;
|
|
|
|
do {
|
|
std::string animation_type = tableData.getStringField("animation_type", "");
|
|
DluAssert(!animation_type.empty());
|
|
AnimationGroupID animationGroupID = tableData.getIntField("animationGroupID", -1);
|
|
DluAssert(animationGroupID != -1);
|
|
|
|
CDAnimation entry;
|
|
entry.animation_name = tableData.getStringField("animation_name", "");
|
|
entry.chance_to_play = tableData.getFloatField("chance_to_play", 1.0f);
|
|
entry.min_loops = tableData.getIntField("min_loops", 0);
|
|
entry.max_loops = tableData.getIntField("max_loops", 0);
|
|
entry.animation_length = tableData.getFloatField("animation_length", 0.0f);
|
|
entry.hideEquip = tableData.getIntField("hideEquip", 0) == 1;
|
|
entry.ignoreUpperBody = tableData.getIntField("ignoreUpperBody", 0) == 1;
|
|
entry.restartable = tableData.getIntField("restartable", 0) == 1;
|
|
entry.face_animation_name = tableData.getStringField("face_animation_name", "");
|
|
entry.priority = tableData.getFloatField("priority", 0.0f);
|
|
entry.blendTime = tableData.getFloatField("blendTime", 0.0f);
|
|
|
|
this->animations[CDAnimationKey(animation_type, animationGroupID)].push_back(entry);
|
|
tableData.nextRow();
|
|
} while (!tableData.eof());
|
|
|
|
tableData.finalize();
|
|
|
|
return true;
|
|
}
|
|
|
|
void CDAnimationsTable::CacheAnimations(const CDAnimationKey animationKey) {
|
|
auto query = CDClientDatabase::CreatePreppedStmt("SELECT * FROM Animations WHERE animationGroupID = ? and animation_type = ?");
|
|
query.bind(1, static_cast<int32_t>(animationKey.second));
|
|
query.bind(2, animationKey.first.c_str());
|
|
// If we received a bad lookup, cache it anyways so we do not run the query again.
|
|
if (!CacheData(query)) {
|
|
this->animations[animationKey];
|
|
}
|
|
}
|
|
|
|
void CDAnimationsTable::CacheAnimationGroup(AnimationGroupID animationGroupID) {
|
|
auto animationEntryCached = this->animations.find(CDAnimationKey("", animationGroupID));
|
|
if (animationEntryCached != this->animations.end()) {
|
|
return;
|
|
}
|
|
|
|
auto query = CDClientDatabase::CreatePreppedStmt("SELECT * FROM Animations WHERE animationGroupID = ?");
|
|
query.bind(1, static_cast<int32_t>(animationGroupID));
|
|
|
|
// Cache the query so we don't run the query again.
|
|
CacheData(query);
|
|
this->animations[CDAnimationKey("", animationGroupID)];
|
|
}
|
|
|
|
CDAnimationLookupResult CDAnimationsTable::GetAnimation(const AnimationID& animationType, const std::string& previousAnimationName, const AnimationGroupID animationGroupID) {
|
|
CDAnimationKey animationKey(animationType, animationGroupID);
|
|
auto randomAnimation = GeneralUtils::GenerateRandomNumber<float>(0, 1);
|
|
auto animationEntryCached = this->animations.find(animationKey);
|
|
if (animationEntryCached == this->animations.end()) {
|
|
this->CacheAnimations(animationKey);
|
|
}
|
|
|
|
auto animationEntry = this->animations.find(animationKey);
|
|
|
|
// If we have only one animation, return it regardless of the chance to play.
|
|
if (animationEntry->second.size() == 1) {
|
|
return CDAnimationLookupResult(animationEntry->second.front());
|
|
}
|
|
|
|
for (auto& animationEntry : animationEntry->second) {
|
|
randomAnimation -= animationEntry.chance_to_play;
|
|
// This is how the client gets the random animation.
|
|
if (animationEntry.animation_name != previousAnimationName && randomAnimation <= 0.0f) return CDAnimationLookupResult(animationEntry);
|
|
}
|
|
|
|
return CDAnimationLookupResult();
|
|
}
|