mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-11-09 01:38:20 +00:00
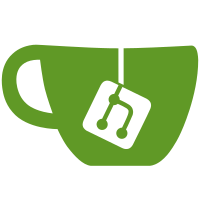
* chore: cleanup LU(W)string writing and add methods for reading remove redunent "packet" from packet reading helpers move write header to bitstreamutils since it's not packet related add tests for reading/writing LU(W)Strings * remove un-needed function defintions in header * make reading and writing more efficient * p p * quotes * remove unneeded default --------- Co-authored-by: David Markowitz <39972741+EmosewaMC@users.noreply.github.com>
122 lines
3.2 KiB
C++
122 lines
3.2 KiB
C++
#include <gtest/gtest.h>
|
|
|
|
#include "dCommonVars.h"
|
|
|
|
TEST(LUString33Test, SerializeWriteTestOld) {
|
|
CBITSTREAM;
|
|
std::string testString;
|
|
for (int i = 0; i < 33; i++) testString += "a";
|
|
for (const auto& c : testString) bitStream.Write(c);
|
|
std::string result;
|
|
char c;
|
|
while (bitStream.Read(c)) result += c;
|
|
ASSERT_EQ(result, testString);
|
|
}
|
|
|
|
TEST(LUString33Test, SerializeWriteTestOldPartial) {
|
|
CBITSTREAM;
|
|
std::string testString;
|
|
for (int i = 0; i < 15; i++) testString += "a";
|
|
for (const auto& c : testString) bitStream.Write(c);
|
|
for (int i = 0; i < 18; i++) bitStream.Write<char>(0);
|
|
std::string result;
|
|
char c;
|
|
int nulls = 18;
|
|
while (bitStream.Read(c)){
|
|
if (c == 0) {
|
|
nulls--;
|
|
continue;
|
|
}
|
|
result += c;
|
|
}
|
|
ASSERT_EQ(nulls, 0);
|
|
ASSERT_EQ(result, testString);
|
|
}
|
|
|
|
TEST(LUString33Test, SerializeWriteTestNew) {
|
|
CBITSTREAM;
|
|
std::string testString;
|
|
for (int i = 0; i < 33; i++) testString += "a";
|
|
bitStream.Write(LUString(testString, 33));
|
|
std::string result;
|
|
char c;
|
|
while (bitStream.Read(c)) result += c;
|
|
ASSERT_EQ(result, testString);
|
|
}
|
|
|
|
TEST(LUString33Test, SerializeWriteTestNewPartial) {
|
|
CBITSTREAM;
|
|
std::string testString;
|
|
for (int i = 0; i < 15; i++) testString += "a";
|
|
bitStream.Write(LUString(testString, 33));
|
|
std::string result;
|
|
char c;
|
|
int nulls = 18;
|
|
while (bitStream.Read(c)){
|
|
if (c == 0) {
|
|
nulls--;
|
|
continue;
|
|
}
|
|
result += c;
|
|
}
|
|
ASSERT_EQ(nulls, 0);
|
|
ASSERT_EQ(result, testString);
|
|
}
|
|
|
|
TEST(LUString33Test, SerializeReadTestOld) {
|
|
CBITSTREAM;
|
|
std::string testString;
|
|
for (int i = 0; i < 33; i++) testString += "a";
|
|
for (const auto& c : testString) bitStream.Write(c);
|
|
std::string result;
|
|
char c;
|
|
while (bitStream.Read(c)) result += c;
|
|
ASSERT_EQ(bitStream.GetNumberOfUnreadBits(), 0);
|
|
ASSERT_EQ(result, testString);
|
|
}
|
|
|
|
TEST(LUString33Test, SerializeReadTestOldPartial) {
|
|
CBITSTREAM;
|
|
std::string testString;
|
|
for (int i = 0; i < 15; i++) testString += "a";
|
|
for (const auto& c : testString) bitStream.Write(c);
|
|
for (int i = 0; i < 18; i++) bitStream.Write<char>(0);
|
|
std::string result;
|
|
char c;
|
|
int nulls = 18;
|
|
while (bitStream.Read(c)){
|
|
if (c == 0) {
|
|
nulls--;
|
|
continue;
|
|
}
|
|
result += c;
|
|
}
|
|
ASSERT_EQ(bitStream.GetNumberOfUnreadBits(), 0);
|
|
ASSERT_EQ(nulls, 0);
|
|
ASSERT_EQ(result, testString);
|
|
}
|
|
|
|
TEST(LUString33Test, SerializeReadTestNew) {
|
|
CBITSTREAM;
|
|
std::string testString;
|
|
for (int i = 0; i < 33; i++) testString += "a";
|
|
bitStream.Write(LUString(testString, 33));
|
|
LUString result(33);
|
|
ASSERT_EQ(result.size, 33);
|
|
ASSERT_TRUE(bitStream.Read(result));
|
|
ASSERT_EQ(bitStream.GetNumberOfUnreadBits(), 0);
|
|
ASSERT_EQ(result.string, testString);
|
|
}
|
|
|
|
TEST(LUString33Test, SerializeReadTestNewPartial) {
|
|
CBITSTREAM;
|
|
std::string testString;
|
|
for (int i = 0; i < 15; i++) testString += "a";
|
|
bitStream.Write(LUString(testString, 33));
|
|
LUString result(33);
|
|
ASSERT_EQ(result.size, 33);
|
|
ASSERT_TRUE(bitStream.Read(result));
|
|
ASSERT_EQ(bitStream.GetNumberOfUnreadBits(), 0);
|
|
ASSERT_EQ(result.string, testString);
|
|
}
|