mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-19 17:11:32 +00:00
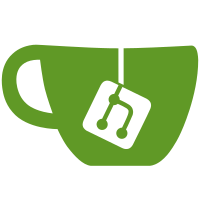
* cast and code cleanup * cast cleanup * bug fixes and improvements * no getBoolField method exists * fixes * unbroke sg cannon scoring * removing comments * Remove the c-style cast warning I added from CMakeLists now that they're gone (it triggers on 3rd party dependencies and slows down compilation) * (Hopefully) fix MacOS compilation error * partially-implemented feedback * more updates to account for feedback * change bool default --------- Co-authored-by: jadebenn <jonahebenn@yahoo.com>
75 lines
2.0 KiB
C++
75 lines
2.0 KiB
C++
#include "PacketUtils.h"
|
|
#include <vector>
|
|
#include <fstream>
|
|
#include "Logger.h"
|
|
#include "Game.h"
|
|
|
|
uint16_t PacketUtils::ReadU16(uint32_t startLoc, Packet* packet) {
|
|
if (startLoc + 2 > packet->length) return 0;
|
|
|
|
std::vector<unsigned char> t;
|
|
for (uint32_t i = startLoc; i < startLoc + 2; i++) t.push_back(packet->data[i]);
|
|
return *(uint16_t*)t.data();
|
|
}
|
|
|
|
uint32_t PacketUtils::ReadU32(uint32_t startLoc, Packet* packet) {
|
|
if (startLoc + 4 > packet->length) return 0;
|
|
|
|
std::vector<unsigned char> t;
|
|
for (uint32_t i = startLoc; i < startLoc + 4; i++) {
|
|
t.push_back(packet->data[i]);
|
|
}
|
|
return *(uint32_t*)t.data();
|
|
}
|
|
|
|
uint64_t PacketUtils::ReadU64(uint32_t startLoc, Packet* packet) {
|
|
if (startLoc + 8 > packet->length) return 0;
|
|
|
|
std::vector<unsigned char> t;
|
|
for (uint32_t i = startLoc; i < startLoc + 8; i++) t.push_back(packet->data[i]);
|
|
return *(uint64_t*)t.data();
|
|
}
|
|
|
|
int64_t PacketUtils::ReadS64(uint32_t startLoc, Packet* packet) {
|
|
if (startLoc + 8 > packet->length) return 0;
|
|
|
|
std::vector<unsigned char> t;
|
|
for (size_t i = startLoc; i < startLoc + 8; i++) t.push_back(packet->data[i]);
|
|
return *(int64_t*)t.data();
|
|
}
|
|
|
|
std::string PacketUtils::ReadString(uint32_t startLoc, Packet* packet, bool wide, uint32_t maxLen) {
|
|
std::string readString = "";
|
|
|
|
if (wide) maxLen *= 2;
|
|
|
|
if (packet->length > startLoc) {
|
|
uint32_t i = 0;
|
|
while (packet->data[startLoc + i] != '\0' && packet->length > static_cast<uint32_t>(startLoc + i) && maxLen > i) {
|
|
readString.push_back(packet->data[startLoc + i]);
|
|
|
|
if (wide) {
|
|
i += 2; // Wide-char string
|
|
} else {
|
|
i++; // Regular string
|
|
}
|
|
}
|
|
}
|
|
|
|
return readString;
|
|
}
|
|
|
|
//! Saves a packet to the filesystem
|
|
void PacketUtils::SavePacket(const std::string& filename, const char* data, size_t length) {
|
|
//If we don't log to the console, don't save the bin files either. This takes up a lot of time.
|
|
if (!Game::logger->GetLogToConsole()) return;
|
|
|
|
std::string path = "packets/" + filename;
|
|
|
|
std::ofstream file(path, std::ios::binary);
|
|
if (!file.is_open()) return;
|
|
|
|
file.write(data, length);
|
|
file.close();
|
|
}
|