mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-11-09 01:38:20 +00:00
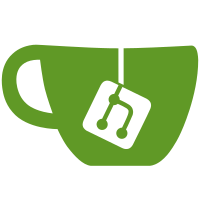
* cast and code cleanup * cast cleanup * bug fixes and improvements * no getBoolField method exists * fixes * unbroke sg cannon scoring * removing comments * Remove the c-style cast warning I added from CMakeLists now that they're gone (it triggers on 3rd party dependencies and slows down compilation) * (Hopefully) fix MacOS compilation error * partially-implemented feedback * more updates to account for feedback * change bool default --------- Co-authored-by: jadebenn <jonahebenn@yahoo.com>
71 lines
1.5 KiB
C++
71 lines
1.5 KiB
C++
#include "ModuleAssemblyComponent.h"
|
|
|
|
ModuleAssemblyComponent::ModuleAssemblyComponent(Entity* parent) : Component(parent) {
|
|
m_SubKey = LWOOBJID_EMPTY;
|
|
m_UseOptionalParts = false;
|
|
m_AssemblyPartsLOTs = u"";
|
|
}
|
|
|
|
ModuleAssemblyComponent::~ModuleAssemblyComponent() {
|
|
|
|
}
|
|
|
|
void ModuleAssemblyComponent::SetSubKey(LWOOBJID value) {
|
|
m_SubKey = value;
|
|
}
|
|
|
|
LWOOBJID ModuleAssemblyComponent::GetSubKey() const {
|
|
return m_SubKey;
|
|
}
|
|
|
|
void ModuleAssemblyComponent::SetUseOptionalParts(bool value) {
|
|
m_UseOptionalParts = value;
|
|
}
|
|
|
|
bool ModuleAssemblyComponent::GetUseOptionalParts() const {
|
|
return m_UseOptionalParts;
|
|
}
|
|
|
|
void ModuleAssemblyComponent::SetAssemblyPartsLOTs(const std::u16string& value) {
|
|
std::u16string val{};
|
|
|
|
val.reserve(value.size() + 1);
|
|
|
|
for (auto character : value) {
|
|
if (character == '+') character = ';';
|
|
|
|
val.push_back(character);
|
|
}
|
|
|
|
val.push_back(';');
|
|
|
|
m_AssemblyPartsLOTs = val;
|
|
}
|
|
|
|
const std::u16string& ModuleAssemblyComponent::GetAssemblyPartsLOTs() const {
|
|
return m_AssemblyPartsLOTs;
|
|
}
|
|
|
|
void ModuleAssemblyComponent::Serialize(RakNet::BitStream* outBitStream, bool bIsInitialUpdate) {
|
|
if (bIsInitialUpdate) {
|
|
outBitStream->Write1();
|
|
|
|
outBitStream->Write(m_SubKey != LWOOBJID_EMPTY);
|
|
if (m_SubKey != LWOOBJID_EMPTY) {
|
|
outBitStream->Write(m_SubKey);
|
|
}
|
|
|
|
outBitStream->Write(m_UseOptionalParts);
|
|
|
|
outBitStream->Write<uint16_t>(m_AssemblyPartsLOTs.size());
|
|
for (char16_t character : m_AssemblyPartsLOTs) {
|
|
outBitStream->Write(character);
|
|
}
|
|
}
|
|
}
|
|
|
|
void ModuleAssemblyComponent::Update(float deltaTime) {
|
|
|
|
}
|
|
|