mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-11-09 01:38:20 +00:00
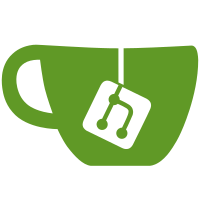
* Logger: Rename logger to Logger from dLogger * Logger: Add compile time filename Fix include issues Add writers Add macros Add macro to force compilation * Logger: Replace calls with macros Allows for filename and line number to be logged * Logger: Add comments and remove extra define Logger: Replace with unique_ptr also flush console at exit. regular file writer should be flushed on file close. Logger: Remove constexpr on variable * Logger: Simplify code * Update Logger.cpp
53 lines
1.4 KiB
C++
53 lines
1.4 KiB
C++
#include "DarkInspirationBehavior.h"
|
|
|
|
#include "BehaviorBranchContext.h"
|
|
#include "Entity.h"
|
|
#include "DestroyableComponent.h"
|
|
#include "EntityManager.h"
|
|
#include "BehaviorContext.h"
|
|
|
|
void DarkInspirationBehavior::Handle(BehaviorContext* context, RakNet::BitStream* bitStream, const BehaviorBranchContext branch) {
|
|
auto* target = Game::entityManager->GetEntity(branch.target);
|
|
|
|
if (target == nullptr) {
|
|
LOG_DEBUG("Failed to find target (%llu)!", branch.target);
|
|
return;
|
|
}
|
|
|
|
auto* destroyableComponent = target->GetComponent<DestroyableComponent>();
|
|
|
|
if (destroyableComponent == nullptr) {
|
|
return;
|
|
}
|
|
|
|
if (destroyableComponent->HasFaction(m_FactionList)) {
|
|
this->m_ActionIfFactionMatches->Handle(context, bitStream, branch);
|
|
}
|
|
}
|
|
|
|
void DarkInspirationBehavior::Calculate(BehaviorContext* context, RakNet::BitStream* bitStream, BehaviorBranchContext branch) {
|
|
auto* target = Game::entityManager->GetEntity(branch.target);
|
|
|
|
if (target == nullptr) {
|
|
LOG_DEBUG("Failed to find target (%llu)!", branch.target);
|
|
|
|
return;
|
|
}
|
|
|
|
auto* destroyableComponent = target->GetComponent<DestroyableComponent>();
|
|
|
|
if (destroyableComponent == nullptr) {
|
|
return;
|
|
}
|
|
|
|
if (destroyableComponent->HasFaction(m_FactionList)) {
|
|
this->m_ActionIfFactionMatches->Calculate(context, bitStream, branch);
|
|
}
|
|
}
|
|
|
|
void DarkInspirationBehavior::Load() {
|
|
this->m_ActionIfFactionMatches = GetAction("action");
|
|
|
|
this->m_FactionList = GetInt("faction_list");
|
|
}
|