mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-11-09 01:38:20 +00:00
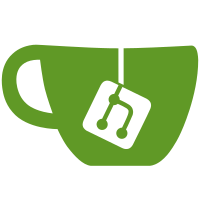
* Logger: Rename logger to Logger from dLogger * Logger: Add compile time filename Fix include issues Add writers Add macros Add macro to force compilation * Logger: Replace calls with macros Allows for filename and line number to be logged * Logger: Add comments and remove extra define Logger: Replace with unique_ptr also flush console at exit. regular file writer should be flushed on file close. Logger: Remove constexpr on variable * Logger: Simplify code * Update Logger.cpp
49 lines
1.3 KiB
C++
49 lines
1.3 KiB
C++
#include "CarBoostBehavior.h"
|
|
#include "BehaviorBranchContext.h"
|
|
#include "GameMessages.h"
|
|
#include "EntityManager.h"
|
|
#include "BehaviorContext.h"
|
|
#include "CharacterComponent.h"
|
|
#include "Game.h"
|
|
#include "Logger.h"
|
|
#include "PossessableComponent.h"
|
|
|
|
void CarBoostBehavior::Handle(BehaviorContext* context, RakNet::BitStream* bitStream, BehaviorBranchContext branch) {
|
|
GameMessages::SendVehicleAddPassiveBoostAction(branch.target, UNASSIGNED_SYSTEM_ADDRESS);
|
|
|
|
auto* entity = Game::entityManager->GetEntity(context->originator);
|
|
|
|
if (entity == nullptr) {
|
|
return;
|
|
}
|
|
|
|
LOG("Activating car boost!");
|
|
|
|
auto* possessableComponent = entity->GetComponent<PossessableComponent>();
|
|
if (possessableComponent != nullptr) {
|
|
|
|
auto* possessor = Game::entityManager->GetEntity(possessableComponent->GetPossessor());
|
|
if (possessor != nullptr) {
|
|
|
|
auto* characterComponent = possessor->GetComponent<CharacterComponent>();
|
|
if (characterComponent != nullptr) {
|
|
LOG("Tracking car boost!");
|
|
characterComponent->UpdatePlayerStatistic(RacingCarBoostsActivated);
|
|
}
|
|
}
|
|
}
|
|
|
|
|
|
m_Action->Handle(context, bitStream, branch);
|
|
|
|
entity->AddCallbackTimer(m_Time, [entity]() {
|
|
GameMessages::SendVehicleRemovePassiveBoostAction(entity->GetObjectID(), UNASSIGNED_SYSTEM_ADDRESS);
|
|
});
|
|
}
|
|
|
|
void CarBoostBehavior::Load() {
|
|
m_Action = GetAction("action");
|
|
|
|
m_Time = GetFloat("time");
|
|
}
|