mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-11-09 01:38:20 +00:00
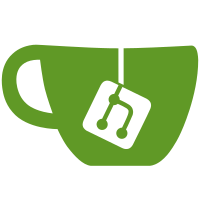
* Logger: Rename logger to Logger from dLogger * Logger: Add compile time filename Fix include issues Add writers Add macros Add macro to force compilation * Logger: Replace calls with macros Allows for filename and line number to be logged * Logger: Add comments and remove extra define Logger: Replace with unique_ptr also flush console at exit. regular file writer should be flushed on file close. Logger: Remove constexpr on variable * Logger: Simplify code * Update Logger.cpp
85 lines
2.3 KiB
C++
85 lines
2.3 KiB
C++
#include "BuffBehavior.h"
|
|
|
|
#include "BehaviorContext.h"
|
|
#include "BehaviorBranchContext.h"
|
|
#include "EntityManager.h"
|
|
#include "Game.h"
|
|
#include "Logger.h"
|
|
#include "DestroyableComponent.h"
|
|
|
|
void BuffBehavior::Handle(BehaviorContext* context, RakNet::BitStream* bitStream, BehaviorBranchContext branch) {
|
|
const auto target = branch.target != LWOOBJID_EMPTY ? branch.target : context->originator;
|
|
|
|
auto* entity = Game::entityManager->GetEntity(target);
|
|
|
|
if (entity == nullptr) {
|
|
LOG("Invalid target (%llu)!", target);
|
|
|
|
return;
|
|
}
|
|
|
|
auto* component = entity->GetComponent<DestroyableComponent>();
|
|
|
|
if (component == nullptr) {
|
|
LOG("Invalid target, no destroyable component (%llu)!", target);
|
|
|
|
return;
|
|
}
|
|
|
|
component->SetMaxHealth(component->GetMaxHealth() + this->m_health);
|
|
component->SetMaxArmor(component->GetMaxArmor() + this->m_armor);
|
|
component->SetMaxImagination(component->GetMaxImagination() + this->m_imagination);
|
|
|
|
Game::entityManager->SerializeEntity(entity);
|
|
|
|
if (!context->unmanaged) {
|
|
if (branch.duration > 0) {
|
|
context->RegisterTimerBehavior(this, branch);
|
|
} else if (branch.start > 0) {
|
|
context->RegisterEndBehavior(this, branch);
|
|
}
|
|
}
|
|
}
|
|
|
|
void BuffBehavior::UnCast(BehaviorContext* context, BehaviorBranchContext branch) {
|
|
const auto target = branch.target != LWOOBJID_EMPTY ? branch.target : context->originator;
|
|
|
|
auto* entity = Game::entityManager->GetEntity(target);
|
|
|
|
if (entity == nullptr) {
|
|
LOG("Invalid target (%llu)!", target);
|
|
|
|
return;
|
|
}
|
|
|
|
auto* component = entity->GetComponent<DestroyableComponent>();
|
|
|
|
if (component == nullptr) {
|
|
LOG("Invalid target, no destroyable component (%llu)!", target);
|
|
|
|
return;
|
|
}
|
|
|
|
component->SetMaxHealth(component->GetMaxHealth() - this->m_health);
|
|
component->SetMaxArmor(component->GetMaxArmor() - this->m_armor);
|
|
component->SetMaxImagination(component->GetMaxImagination() - this->m_imagination);
|
|
|
|
Game::entityManager->SerializeEntity(entity);
|
|
}
|
|
|
|
void BuffBehavior::Timer(BehaviorContext* context, const BehaviorBranchContext branch, LWOOBJID second) {
|
|
UnCast(context, branch);
|
|
}
|
|
|
|
void BuffBehavior::End(BehaviorContext* context, const BehaviorBranchContext branch, LWOOBJID second) {
|
|
UnCast(context, branch);
|
|
}
|
|
|
|
void BuffBehavior::Load() {
|
|
this->m_health = GetInt("life");
|
|
|
|
this->m_armor = GetInt("armor");
|
|
|
|
this->m_imagination = GetInt("imag");
|
|
}
|