mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-11-08 17:28:20 +00:00
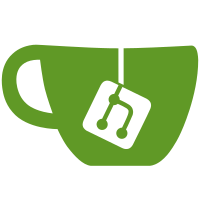
* Move away from constructor queries Fix up other large tables to have proper backup lookups Revert "idk im just dumb ig" This reverts commit 5d5be5df53b8959b42b291613d7db749a65a3585. idk im just dumb ig * Fix slow components registry lookup * add define for cdclient cache all * Huge loot namespace rework - Remove all excess memory usage - do not cache components registry - cache loot matrices on startup of the destroyable component - convert loot singleton class to a namespace - rework loot cdclient tables to operate closer to how someone would actually use them (basically doing the previous LootGenerator::LootGenerator caching but in those tables) - Memory usage reduced by 10%+ across the board * cache rebuild matrix * Database: move reading to own function Also change name of cache to PascalCase * Database: Move common function rading
48 lines
1.5 KiB
C++
48 lines
1.5 KiB
C++
#include "ScriptedPowerupSpawner.h"
|
|
#include "RenderComponent.h"
|
|
#include "EntityManager.h"
|
|
#include "Loot.h"
|
|
|
|
void ScriptedPowerupSpawner::OnTemplateStartup(Entity* self) {
|
|
self->SetVar<uint32_t>(u"currentCycle", 1);
|
|
self->AddTimer("timeToSpawn", self->GetVar<float_t>(u"delayToFirstCycle"));
|
|
}
|
|
|
|
void ScriptedPowerupSpawner::OnTimerDone(Entity* self, std::string message) {
|
|
if (message == "die") {
|
|
self->Smash();
|
|
} else if (message == "timeToSpawn") {
|
|
|
|
const auto itemLOT = self->GetVar<LOT>(u"lootLOT");
|
|
|
|
// Build drop table
|
|
std::unordered_map<LOT, int32_t> drops;
|
|
|
|
drops.emplace(itemLOT, 1);
|
|
|
|
// Spawn the required number of powerups
|
|
auto* owner = Game::entityManager->GetEntity(self->GetSpawnerID());
|
|
if (owner != nullptr) {
|
|
auto* renderComponent = self->GetComponent<RenderComponent>();
|
|
for (auto i = 0; i < self->GetVar<uint32_t>(u"numberOfPowerups"); i++) {
|
|
if (renderComponent != nullptr) {
|
|
renderComponent->PlayEffect(0, u"cast", "N_cast");
|
|
}
|
|
|
|
Loot::DropLoot(owner, self, drops, 0, 0);
|
|
}
|
|
|
|
// Increment the current cycle
|
|
if (self->GetVar<uint32_t>(u"currentCycle") < self->GetVar<uint32_t>(u"numCycles")) {
|
|
self->AddTimer("timeToSpawn", self->GetVar<float_t>(u"secPerCycle"));
|
|
self->SetVar<uint32_t>(u"currentCycle", self->GetVar<uint32_t>(u"currentCycle") + 1);
|
|
}
|
|
|
|
// Kill if this was the last cycle
|
|
if (self->GetVar<uint32_t>(u"currentCycle") >= self->GetVar<uint32_t>(u"numCycles")) {
|
|
self->AddTimer("die", self->GetVar<float_t>(u"deathDelay"));
|
|
}
|
|
}
|
|
}
|
|
}
|