mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-11-09 01:38:20 +00:00
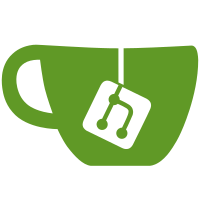
* allow usage of NiPoint3 and NiQuaternion in constexpr context * removed .cpp files entirely * moving circular dependency circumvention stuff to an .inl file * real world usage!!!!! * reverting weird branch cross-pollination * removing more weird branch cross-pollination * remove comment * added inverse header guard to inl file * Update NiPoint3.inl * trying different constructor syntax * reorganize into .inl files for readability * uncomment include * moved non-constexpr definitions to cpp file * moved static definitions back to inl files * testing fix * moved constants into seperate namespace * Undo change in build-and-test.yml * nodiscard
71 lines
1.7 KiB
C++
71 lines
1.7 KiB
C++
#include "BuildBorderComponent.h"
|
|
|
|
#include "EntityManager.h"
|
|
#include "GameMessages.h"
|
|
#include "Entity.h"
|
|
#include "Game.h"
|
|
#include "Logger.h"
|
|
#include "InventoryComponent.h"
|
|
#include "Item.h"
|
|
#include "PropertyManagementComponent.h"
|
|
|
|
BuildBorderComponent::BuildBorderComponent(Entity* parent) : Component(parent) {
|
|
}
|
|
|
|
BuildBorderComponent::~BuildBorderComponent() {
|
|
}
|
|
|
|
void BuildBorderComponent::OnUse(Entity* originator) {
|
|
if (originator->GetCharacter()) {
|
|
const auto& entities = Game::entityManager->GetEntitiesInGroup("PropertyPlaque");
|
|
|
|
auto buildArea = m_Parent->GetObjectID();
|
|
|
|
if (!entities.empty()) {
|
|
buildArea = entities[0]->GetObjectID();
|
|
|
|
LOG("Using PropertyPlaque");
|
|
}
|
|
|
|
auto* inventoryComponent = originator->GetComponent<InventoryComponent>();
|
|
|
|
if (inventoryComponent == nullptr) {
|
|
return;
|
|
}
|
|
|
|
auto* thinkingHat = inventoryComponent->FindItemByLot(6086);
|
|
|
|
if (thinkingHat == nullptr) {
|
|
return;
|
|
}
|
|
|
|
inventoryComponent->PushEquippedItems();
|
|
|
|
LOG("Starting with %llu", buildArea);
|
|
|
|
if (PropertyManagementComponent::Instance() != nullptr) {
|
|
GameMessages::SendStartArrangingWithItem(
|
|
originator,
|
|
originator->GetSystemAddress(),
|
|
true,
|
|
buildArea,
|
|
originator->GetPosition(),
|
|
0,
|
|
thinkingHat->GetId(),
|
|
thinkingHat->GetLot(),
|
|
4,
|
|
0,
|
|
-1,
|
|
NiPoint3Constant::ZERO,
|
|
0
|
|
);
|
|
} else {
|
|
GameMessages::SendStartArrangingWithItem(originator, originator->GetSystemAddress(), true, buildArea, originator->GetPosition());
|
|
}
|
|
|
|
InventoryComponent* inv = m_Parent->GetComponent<InventoryComponent>();
|
|
if (!inv) return;
|
|
inv->PushEquippedItems(); // technically this is supposed to happen automatically... but it doesnt? so just keep this here
|
|
}
|
|
}
|