mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2025-07-05 19:20:01 +00:00
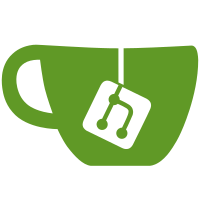
* CDClient cleanup and optimization - Use static function to get table name - Remove unused GetName function - Replace above function with a static GetTableName function - Remove verbose comments - Remove verbose initializers - Remove need to specify table name when getting a table by name - Remove unused typedef for mac and linux * Re-add unused table Convert tables to singletons - Convert all CDClient tables to singletons - Move Singleton.h to dCommon - Reduce header clutter in CDClientManager
70 lines
2.4 KiB
C++
70 lines
2.4 KiB
C++
#include "QbEnemyStunner.h"
|
|
#include "SkillComponent.h"
|
|
#include "CDClientManager.h"
|
|
#include "DestroyableComponent.h"
|
|
|
|
#include "CDObjectSkillsTable.h"
|
|
#include "CDSkillBehaviorTable.h"
|
|
|
|
void QbEnemyStunner::OnRebuildComplete(Entity* self, Entity* target) {
|
|
auto* destroyable = self->GetComponent<DestroyableComponent>();
|
|
|
|
if (destroyable != nullptr) {
|
|
destroyable->SetFaction(115);
|
|
}
|
|
|
|
auto skillComponent = self->GetComponent<SkillComponent>();
|
|
if (!skillComponent) return;
|
|
|
|
// Get the skill IDs of this object.
|
|
CDObjectSkillsTable* skillsTable = CDClientManager::Instance().GetTable<CDObjectSkillsTable>();
|
|
auto skills = skillsTable->Query([=](CDObjectSkills entry) {return (entry.objectTemplate == self->GetLOT()); });
|
|
std::map<uint32_t, uint32_t> skillBehaviorMap;
|
|
// For each skill, cast it with the associated behavior ID.
|
|
for (auto skill : skills) {
|
|
CDSkillBehaviorTable* skillBehaviorTable = CDClientManager::Instance().GetTable<CDSkillBehaviorTable>();
|
|
CDSkillBehavior behaviorData = skillBehaviorTable->GetSkillByID(skill.skillID);
|
|
|
|
skillBehaviorMap.insert(std::make_pair(skill.skillID, behaviorData.behaviorID));
|
|
}
|
|
|
|
// If there are no skills found, insert a default skill to use.
|
|
if (skillBehaviorMap.size() == 0) {
|
|
skillBehaviorMap.insert(std::make_pair(499U, 6095U));
|
|
}
|
|
|
|
// Start all skills associated with the object next tick
|
|
self->AddTimer("TickTime", 0);
|
|
|
|
self->AddTimer("PlayEffect", 20);
|
|
|
|
self->SetVar<std::map<uint32_t, uint32_t>>(u"skillBehaviorMap", skillBehaviorMap);
|
|
}
|
|
|
|
void QbEnemyStunner::OnTimerDone(Entity* self, std::string timerName) {
|
|
if (timerName == "DieTime") {
|
|
self->Smash();
|
|
|
|
self->CancelAllTimers();
|
|
} else if (timerName == "PlayEffect") {
|
|
self->SetNetworkVar(u"startEffect", 5.0f, UNASSIGNED_SYSTEM_ADDRESS);
|
|
|
|
self->AddTimer("DieTime", 5.0f);
|
|
} else if (timerName == "TickTime") {
|
|
auto* skillComponent = self->GetComponent<SkillComponent>();
|
|
|
|
if (skillComponent != nullptr) {
|
|
auto skillBehaviorMap = self->GetVar<std::map<uint32_t, uint32_t>>(u"skillBehaviorMap");
|
|
if (skillBehaviorMap.size() == 0) {
|
|
// Should no skills have been found, default to the mermaid stunner
|
|
skillComponent->CalculateBehavior(499U, 6095U, LWOOBJID_EMPTY);
|
|
} else {
|
|
for (auto pair : skillBehaviorMap) {
|
|
skillComponent->CalculateBehavior(pair.first, pair.second, LWOOBJID_EMPTY);
|
|
}
|
|
}
|
|
}
|
|
self->AddTimer("TickTime", 1);
|
|
}
|
|
}
|