mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-19 17:11:32 +00:00
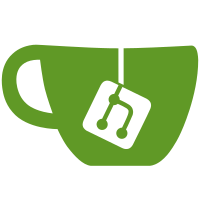
* Add automatic migrations for CDServer Add support to automatically migrate and update CDServers with new migrations. Also adds support to simplify the setup process by simply putting the fdb in the res folder and letting the server convert it to sqlite. This reduces the amount of back and forth when setting up a server. * Remove transaction language * Add DML execution `poggers` Add a way to execute DML commands through the sqlite connection on the server. * Make DML Commands more robust On the off chance the server is shutdown before the whole migration is run, lets just not add it to our "finished list" until the whole file is done. * Update README
58 lines
1.3 KiB
C++
58 lines
1.3 KiB
C++
#pragma once
|
|
|
|
// C++
|
|
#include <string>
|
|
|
|
// SQLite
|
|
#include "CppSQLite3.h"
|
|
|
|
/*
|
|
* Optimization settings
|
|
*/
|
|
|
|
#include <sstream>
|
|
#include <iostream>
|
|
|
|
// Enable this to cache all entries in each table for fast access, comes with more memory cost
|
|
//#define CDCLIENT_CACHE_ALL
|
|
|
|
// Enable this to skip some unused columns in some tables
|
|
#define UNUSED(v)
|
|
|
|
/*!
|
|
\file CDClientDatabase.hpp
|
|
\brief An interface between the CDClient.sqlite file and the server
|
|
*/
|
|
|
|
//! The CDClient Database namespace
|
|
namespace CDClientDatabase {
|
|
|
|
//! Opens a connection with the CDClient
|
|
/*!
|
|
\param filename The filename
|
|
*/
|
|
void Connect(const std::string& filename);
|
|
|
|
//! Queries the CDClient
|
|
/*!
|
|
\param query The query
|
|
\return The results of the query
|
|
*/
|
|
CppSQLite3Query ExecuteQuery(const std::string& query);
|
|
|
|
//! Updates the CDClient file with Data Manipulation Language (DML) commands.
|
|
/*!
|
|
\param query The DML command to run. DML command can be multiple queries in one string but only
|
|
the last one will return its number of updated rows.
|
|
\return The number of updated rows.
|
|
*/
|
|
int ExecuteDML(const std::string& query);
|
|
|
|
//! Queries the CDClient and parses arguments
|
|
/*!
|
|
\param query The query with formatted arguments
|
|
\return prepared SQLite Statement
|
|
*/
|
|
CppSQLite3Statement CreatePreppedStmt(const std::string& query);
|
|
};
|