mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2025-04-26 16:46:31 +00:00
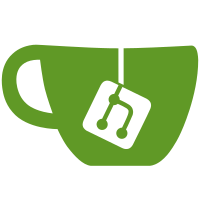
* feat: broadcast achievements in chat as in live Tested that everyone on the receiving players' friends list receives the announcement as it went out in live. Only works for achievements that have an entry in the MissionEmail table. This may have been sent out to everyone in your zone as well however we don't really have a way to verify this aside from questioning why the client checks for the receiver being in the ignore list. This is the only hint to me that this may have been broadcast to more than friends but again, no proof. * Add initial response msg and sending * Revert "Add initial response msg and sending" This reverts commit fb942e4692747ff1debea2e0ad00d22dd0d632f3.
55 lines
1.5 KiB
C++
55 lines
1.5 KiB
C++
/*
|
|
* Darkflame Universe
|
|
* Copyright 2018
|
|
*/
|
|
|
|
#ifndef CHATPACKETS_H
|
|
#define CHATPACKETS_H
|
|
|
|
struct SystemAddress;
|
|
|
|
#include <string>
|
|
#include "dCommonVars.h"
|
|
#include "MessageType/Chat.h"
|
|
#include "BitStreamUtils.h"
|
|
|
|
struct ShowAllRequest{
|
|
LWOOBJID requestor = LWOOBJID_EMPTY;
|
|
bool displayZoneData = true;
|
|
bool displayIndividualPlayers = true;
|
|
void Serialize(RakNet::BitStream& bitStream);
|
|
void Deserialize(RakNet::BitStream& inStream);
|
|
};
|
|
|
|
struct FindPlayerRequest{
|
|
LWOOBJID requestor = LWOOBJID_EMPTY;
|
|
LUWString playerName;
|
|
void Serialize(RakNet::BitStream& bitStream);
|
|
void Deserialize(RakNet::BitStream& inStream);
|
|
};
|
|
|
|
namespace ChatPackets {
|
|
|
|
struct Announcement {
|
|
std::string title;
|
|
std::string message;
|
|
void Send();
|
|
};
|
|
|
|
struct AchievementNotify : public LUBitStream {
|
|
LUWString targetPlayerName{};
|
|
uint32_t missionEmailID{};
|
|
LWOOBJID earningPlayerID{};
|
|
LUWString earnerName{};
|
|
AchievementNotify() : LUBitStream(eConnectionType::CHAT, MessageType::Chat::ACHIEVEMENT_NOTIFY) {}
|
|
void Serialize(RakNet::BitStream& bitstream) const override;
|
|
bool Deserialize(RakNet::BitStream& bitstream) override;
|
|
};
|
|
|
|
void SendChatMessage(const SystemAddress& sysAddr, char chatChannel, const std::string& senderName, LWOOBJID playerObjectID, bool senderMythran, const std::u16string& message);
|
|
void SendSystemMessage(const SystemAddress& sysAddr, const std::u16string& message, bool broadcast = false);
|
|
void SendMessageFail(const SystemAddress& sysAddr);
|
|
};
|
|
|
|
#endif // CHATPACKETS_H
|