mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2025-04-26 16:46:31 +00:00
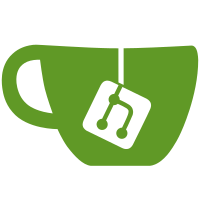
* feat: broadcast achievements in chat as in live Tested that everyone on the receiving players' friends list receives the announcement as it went out in live. Only works for achievements that have an entry in the MissionEmail table. This may have been sent out to everyone in your zone as well however we don't really have a way to verify this aside from questioning why the client checks for the receiver being in the ignore list. This is the only hint to me that this may have been broadcast to more than friends but again, no proof. * Add initial response msg and sending * Revert "Add initial response msg and sending" This reverts commit fb942e4692747ff1debea2e0ad00d22dd0d632f3.
135 lines
4.3 KiB
C++
135 lines
4.3 KiB
C++
/*
|
|
* Darkflame Universe
|
|
* Copyright 2018
|
|
*/
|
|
|
|
#include "ChatPackets.h"
|
|
#include "RakNetTypes.h"
|
|
#include "BitStream.h"
|
|
#include "Game.h"
|
|
#include "BitStreamUtils.h"
|
|
#include "dServer.h"
|
|
#include "eConnectionType.h"
|
|
#include "MessageType/Chat.h"
|
|
|
|
void ShowAllRequest::Serialize(RakNet::BitStream& bitStream) {
|
|
BitStreamUtils::WriteHeader(bitStream, eConnectionType::CHAT, MessageType::Chat::SHOW_ALL);
|
|
bitStream.Write(this->requestor);
|
|
bitStream.Write(this->displayZoneData);
|
|
bitStream.Write(this->displayIndividualPlayers);
|
|
}
|
|
|
|
void ShowAllRequest::Deserialize(RakNet::BitStream& inStream) {
|
|
inStream.Read(this->requestor);
|
|
inStream.Read(this->displayZoneData);
|
|
inStream.Read(this->displayIndividualPlayers);
|
|
}
|
|
|
|
void FindPlayerRequest::Serialize(RakNet::BitStream& bitStream) {
|
|
BitStreamUtils::WriteHeader(bitStream, eConnectionType::CHAT, MessageType::Chat::WHO);
|
|
bitStream.Write(this->requestor);
|
|
bitStream.Write(this->playerName);
|
|
}
|
|
|
|
void FindPlayerRequest::Deserialize(RakNet::BitStream& inStream) {
|
|
inStream.Read(this->requestor);
|
|
inStream.Read(this->playerName);
|
|
}
|
|
|
|
void ChatPackets::SendChatMessage(const SystemAddress& sysAddr, char chatChannel, const std::string& senderName, LWOOBJID playerObjectID, bool senderMythran, const std::u16string& message) {
|
|
CBITSTREAM;
|
|
BitStreamUtils::WriteHeader(bitStream, eConnectionType::CHAT, MessageType::Chat::GENERAL_CHAT_MESSAGE);
|
|
|
|
bitStream.Write<uint64_t>(0);
|
|
bitStream.Write(chatChannel);
|
|
|
|
bitStream.Write<uint32_t>(message.size());
|
|
bitStream.Write(LUWString(senderName));
|
|
|
|
bitStream.Write(playerObjectID);
|
|
bitStream.Write<uint16_t>(0);
|
|
bitStream.Write<char>(0);
|
|
|
|
for (uint32_t i = 0; i < message.size(); ++i) {
|
|
bitStream.Write<uint16_t>(message[i]);
|
|
}
|
|
bitStream.Write<uint16_t>(0);
|
|
|
|
SEND_PACKET_BROADCAST;
|
|
}
|
|
|
|
void ChatPackets::SendSystemMessage(const SystemAddress& sysAddr, const std::u16string& message, const bool broadcast) {
|
|
CBITSTREAM;
|
|
BitStreamUtils::WriteHeader(bitStream, eConnectionType::CHAT, MessageType::Chat::GENERAL_CHAT_MESSAGE);
|
|
|
|
bitStream.Write<uint64_t>(0);
|
|
bitStream.Write<char>(4);
|
|
|
|
bitStream.Write<uint32_t>(message.size());
|
|
bitStream.Write(LUWString("", 33));
|
|
|
|
bitStream.Write<uint64_t>(0);
|
|
bitStream.Write<uint16_t>(0);
|
|
bitStream.Write<char>(0);
|
|
|
|
for (uint32_t i = 0; i < message.size(); ++i) {
|
|
bitStream.Write<uint16_t>(message[i]);
|
|
}
|
|
|
|
bitStream.Write<uint16_t>(0);
|
|
|
|
//This is so Wincent's announcement works:
|
|
if (sysAddr != UNASSIGNED_SYSTEM_ADDRESS) {
|
|
SEND_PACKET;
|
|
return;
|
|
}
|
|
|
|
SEND_PACKET_BROADCAST;
|
|
}
|
|
|
|
void ChatPackets::SendMessageFail(const SystemAddress& sysAddr) {
|
|
//0x00 - "Chat is currently disabled."
|
|
//0x01 - "Upgrade to a full LEGO Universe Membership to chat with other players."
|
|
|
|
CBITSTREAM;
|
|
BitStreamUtils::WriteHeader(bitStream, eConnectionType::CLIENT, MessageType::Client::SEND_CANNED_TEXT);
|
|
bitStream.Write<uint8_t>(0); //response type, options above ^
|
|
//docs say there's a wstring here-- no idea what it's for, or if it's even needed so leaving it as is for now.
|
|
SEND_PACKET;
|
|
}
|
|
|
|
void ChatPackets::Announcement::Send() {
|
|
CBITSTREAM;
|
|
BitStreamUtils::WriteHeader(bitStream, eConnectionType::CHAT, MessageType::Chat::GM_ANNOUNCE);
|
|
bitStream.Write<uint32_t>(title.size());
|
|
bitStream.Write(title);
|
|
bitStream.Write<uint32_t>(message.size());
|
|
bitStream.Write(message);
|
|
SEND_PACKET_BROADCAST;
|
|
}
|
|
|
|
void ChatPackets::AchievementNotify::Serialize(RakNet::BitStream& bitstream) const {
|
|
bitstream.Write<uint64_t>(0); // Packing
|
|
bitstream.Write<uint32_t>(0); // Packing
|
|
bitstream.Write<uint8_t>(0); // Packing
|
|
bitstream.Write(targetPlayerName);
|
|
bitstream.Write<uint64_t>(0); // Packing / No way to know meaning because of not enough data.
|
|
bitstream.Write<uint32_t>(0); // Packing / No way to know meaning because of not enough data.
|
|
bitstream.Write<uint16_t>(0); // Packing / No way to know meaning because of not enough data.
|
|
bitstream.Write<uint8_t>(0); // Packing / No way to know meaning because of not enough data.
|
|
bitstream.Write(missionEmailID);
|
|
bitstream.Write(earningPlayerID);
|
|
bitstream.Write(earnerName);
|
|
}
|
|
|
|
bool ChatPackets::AchievementNotify::Deserialize(RakNet::BitStream& bitstream) {
|
|
bitstream.IgnoreBytes(13);
|
|
VALIDATE_READ(bitstream.Read(targetPlayerName));
|
|
bitstream.IgnoreBytes(15);
|
|
VALIDATE_READ(bitstream.Read(missionEmailID));
|
|
VALIDATE_READ(bitstream.Read(earningPlayerID));
|
|
VALIDATE_READ(bitstream.Read(earnerName));
|
|
|
|
return true;
|
|
}
|