mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-19 17:11:32 +00:00
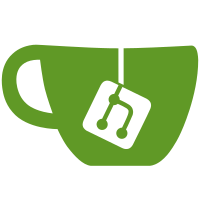
* Logger: Rename logger to Logger from dLogger * Logger: Add compile time filename Fix include issues Add writers Add macros Add macro to force compilation * Logger: Replace calls with macros Allows for filename and line number to be logged * Logger: Add comments and remove extra define Logger: Replace with unique_ptr also flush console at exit. regular file writer should be flushed on file close. Logger: Remove constexpr on variable * Logger: Simplify code * Update Logger.cpp
45 lines
1.1 KiB
C++
45 lines
1.1 KiB
C++
#include "TauntBehavior.h"
|
|
#include "BehaviorBranchContext.h"
|
|
#include "BehaviorContext.h"
|
|
#include "BaseCombatAIComponent.h"
|
|
#include "EntityManager.h"
|
|
#include "Logger.h"
|
|
|
|
|
|
void TauntBehavior::Handle(BehaviorContext* context, RakNet::BitStream* bitStream, BehaviorBranchContext branch) {
|
|
auto* target = Game::entityManager->GetEntity(branch.target);
|
|
|
|
if (target == nullptr) {
|
|
LOG("Failed to find target (%llu)!", branch.target);
|
|
|
|
return;
|
|
}
|
|
|
|
auto* combatComponent = target->GetComponent<BaseCombatAIComponent>();
|
|
|
|
if (combatComponent != nullptr) {
|
|
combatComponent->Taunt(context->originator, m_threatToAdd);
|
|
}
|
|
}
|
|
|
|
void TauntBehavior::Calculate(BehaviorContext* context, RakNet::BitStream* bitStream, BehaviorBranchContext branch) {
|
|
auto* target = Game::entityManager->GetEntity(branch.target);
|
|
|
|
if (target == nullptr) {
|
|
LOG("Failed to find target (%llu)!", branch.target);
|
|
|
|
return;
|
|
}
|
|
|
|
auto* combatComponent = target->GetComponent<BaseCombatAIComponent>();
|
|
|
|
if (combatComponent != nullptr) {
|
|
combatComponent->Taunt(context->originator, m_threatToAdd);
|
|
}
|
|
}
|
|
|
|
void TauntBehavior::Load() {
|
|
this->m_threatToAdd = GetFloat("threat to add");
|
|
}
|
|
|