mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-19 17:11:32 +00:00
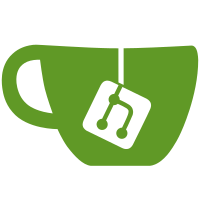
* Move EntityManager to Game namespace * move initialization to later Need to wait for dZoneManager to be initialized. * Fix bugs - Cannot delete from a RandomAccessIterator while in a range based for loop. Touchup zone manager initialize replace magic numbers with better named constants replace magic zonecontrol id with a more readable hex alternative condense stack variables move initializers closer to their use initialize entity manager with zone control change initialize timings If zone is not zero we expect to initialize the entity manager during zone manager initialization Add constexpr for zone control LOT * Add proper error handling * revert vanity changes * Update WorldServer.cpp * Update dZoneManager.cpp
39 lines
1.3 KiB
C++
39 lines
1.3 KiB
C++
#include "LootBuffBehavior.h"
|
|
|
|
void LootBuffBehavior::Handle(BehaviorContext* context, RakNet::BitStream* bitStream, BehaviorBranchContext branch) {
|
|
auto target = Game::entityManager->GetEntity(context->caster);
|
|
if (!target) return;
|
|
|
|
auto controllablePhysicsComponent = target->GetComponent<ControllablePhysicsComponent>();
|
|
if (!controllablePhysicsComponent) return;
|
|
|
|
controllablePhysicsComponent->AddPickupRadiusScale(m_Scale);
|
|
Game::entityManager->SerializeEntity(target);
|
|
|
|
if (branch.duration > 0) context->RegisterTimerBehavior(this, branch);
|
|
|
|
}
|
|
|
|
void LootBuffBehavior::Calculate(BehaviorContext* context, RakNet::BitStream* bitStream, BehaviorBranchContext branch) {
|
|
Handle(context, bitStream, branch);
|
|
}
|
|
|
|
void LootBuffBehavior::UnCast(BehaviorContext* context, BehaviorBranchContext branch) {
|
|
auto target = Game::entityManager->GetEntity(context->caster);
|
|
if (!target) return;
|
|
|
|
auto controllablePhysicsComponent = target->GetComponent<ControllablePhysicsComponent>();
|
|
if (!controllablePhysicsComponent) return;
|
|
|
|
controllablePhysicsComponent->RemovePickupRadiusScale(m_Scale);
|
|
Game::entityManager->SerializeEntity(target);
|
|
}
|
|
|
|
void LootBuffBehavior::Timer(BehaviorContext* context, BehaviorBranchContext branch, LWOOBJID second) {
|
|
UnCast(context, branch);
|
|
}
|
|
|
|
void LootBuffBehavior::Load() {
|
|
this->m_Scale = GetFloat("scale");
|
|
}
|