mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-11-13 19:58:21 +00:00
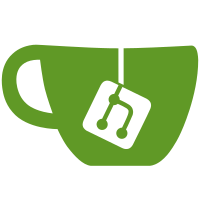
add final missing scripts for nt also fix the turnin for the breadcrumb missions not showing the completion window. Fix another missing script Add another script fix include guards Fix dirt clouds not appearing on mission accept
92 lines
2.2 KiB
C++
92 lines
2.2 KiB
C++
/*
|
|
* Darkflame Universe
|
|
* Copyright 2019
|
|
*/
|
|
|
|
#ifndef MISSIONOFFERCOMPONENT_H
|
|
#define MISSIONOFFERCOMPONENT_H
|
|
|
|
#include "dCommonVars.h"
|
|
#include "Component.h"
|
|
#include <vector>
|
|
#include <stdint.h>
|
|
#include "eReplicaComponentType.h"
|
|
|
|
class Entity;
|
|
|
|
/**
|
|
* Light wrapper around missions that may be offered by an entity
|
|
*/
|
|
struct OfferedMission {
|
|
OfferedMission(uint32_t missionId, bool offersMission, bool acceptsMission);
|
|
|
|
/**
|
|
* Returns the ID of the mission
|
|
* @return the ID of the mission
|
|
*/
|
|
uint32_t GetMissionId() const;
|
|
|
|
/**
|
|
* Returns if this mission is offered by the entity
|
|
* @return true if this mission is offered by the entity, false otherwise
|
|
*/
|
|
bool GetOffersMission() const;
|
|
|
|
/**
|
|
* Returns if this mission may be accepted by the entity (currently unused)
|
|
* @return true if this mission may be accepted by the entity, false otherwise
|
|
*/
|
|
bool GetAcceptsMission() const;
|
|
|
|
private:
|
|
|
|
/**
|
|
* The ID of the mission
|
|
*/
|
|
uint32_t missionId;
|
|
|
|
/**
|
|
* Determines if the mission is offered by the entity
|
|
*/
|
|
bool offersMission;
|
|
|
|
/**
|
|
* Determines if the mission can be accepted by the entity
|
|
*/
|
|
bool acceptsMission;
|
|
};
|
|
|
|
/**
|
|
* Allows entities to offer missions to other entities, depending on their mission inventory progression.
|
|
*/
|
|
class MissionOfferComponent : public Component {
|
|
public:
|
|
inline static const eReplicaComponentType ComponentType = eReplicaComponentType::MISSION_OFFER;
|
|
|
|
MissionOfferComponent(Entity* parent, LOT parentLot);
|
|
~MissionOfferComponent() override;
|
|
|
|
/**
|
|
* Handles the OnUse event triggered by some entity, determines which missions to show based on what they may
|
|
* hand in now and what they may start based on their mission history.
|
|
* @param originator the entity that triggered the event
|
|
*/
|
|
void OnUse(Entity* originator) override;
|
|
|
|
/**
|
|
* Offers all the missions an entity can accept to said entity
|
|
* @param entity the entity to offer missions to
|
|
* @param specifiedMissionId optional mission ID if you wish to offer a specific mission
|
|
*/
|
|
void OfferMissions(Entity* entity, uint32_t specifiedMissionId = 0);
|
|
|
|
private:
|
|
|
|
/**
|
|
* The missions this entity has to offer
|
|
*/
|
|
std::vector<OfferedMission*> offeredMissions;
|
|
};
|
|
|
|
#endif // MISSIONOFFERCOMPONENT_H
|