mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2025-07-03 10:09:54 +00:00
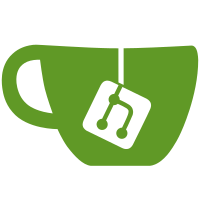
* Add MSVC optimization flags * test moving flags to json * Update CMakePresets.json * testing * trying more variations on the flags * third test * testing if these even have any effect * ditto * final(?) try for now * ONE MORE TIME * trying 'init' flags instead * export the compile commands so I can see if they're having any effect * move out g++ O2 flag * add Linux debug preset * update CMake presets * edit macos presets * try adding build types back to mac * macos refuses to work :( * try using compiler flags for mac instead * fix typo in windows preset * build reorganization and experimental clang support * temporarily remove macos build for testing purposes * updated cmake workflows * unexclude toolchain dir * update .gitignore * fix build directory issue * edit build script * update cmake configs * attempted docker fix * try zero-initializinng this struct to solve docker issue * try fixing macos build * one last MacOS try for the night * try disabling an apple-specific build rule * more fiddling with mac test builds * try and narrow down the macos build failure cause * try stripping out all the custom macos test logic again * I'm really just throwing everything to the wall and seeing what sticks * more macos tinkering * implib * try manual link directory specification * save me * aaaaaaaaa * paths paths paths * Revert "paths paths paths" This reverts commit 9a7d86aa6c59e73de27fbcda2111f7a1472008f4. * Revert "aaaaaaaaa" This reverts commit 338279c396e7c4a78174929a0aaf5205f2c026e6. * Revert "save me" This reverts commit bd73aa21a9cd1625f7cf567ab5b56bde46c0af0e. * Revert "try manual link directory specification" This reverts commit 0c2d40632ee5df9c241532d8bf62de9969e47f51. * Revert "implib" This reverts commit d41349d6edada6a041c64971730eed1c51af14c5. * Revert "more macos tinkering" This reverts commit 829ec35b57983ad4444d90ab780fff95a8b47608. * Revert "I'm really just throwing everything to the wall and seeing what sticks" This reverts commit 1a05b027fe822a94e5a6b70e6c744623d6a98e61. * Revert "try stripping out all the custom macos test logic again" This reverts commit cc15a26ce80ff9cfec5f1a94b0c00c42e1832c55. * Revert "try and narrow down the macos build failure cause" This reverts commit 5fd86833fa6e421860496c3626415ab70c93a795. * Revert "more fiddling with mac test builds" This reverts commit 0f843c02c90b2aa5f0c211e19c47b00798c295c8. * Revert "try disabling an apple-specific build rule" This reverts commit 45ec66e97605e3ea5b0a76f6eed0ec6f955c1675. * back to debug messages * see if this re-breaks mac * are these messages actually somehow fixing the issue? * was not actually fixed * add debug messages (again) * debug try 2 * change runtime output dir * rename gcc to gnu * expand cmake presets * fix preset * change defaults * altered cmake configuration scripts * disable /WX on MSVC * update github actions * update build presets * change gnu and clang build directories to enable consistent artifact generation * add RelWithDebInfo presets and move -Werror flag into presets.json * use DLU_CONFIG_DIR envvar * CMakePresets indentation * temp fix for MSVC debug builds
107 lines
3.7 KiB
C++
107 lines
3.7 KiB
C++
#include <gtest/gtest.h>
|
|
|
|
#include <vector>
|
|
|
|
#include "Amf3.h"
|
|
|
|
TEST(dCommonTests, AMF3AssociativeArrayTest) {
|
|
|
|
AMFArrayValue array;
|
|
array.Insert("true", true);
|
|
array.Insert("false", false);
|
|
|
|
// test associative can insert values
|
|
ASSERT_EQ(array.GetAssociative().size(), 2);
|
|
ASSERT_EQ(array.Get<bool>("true")->GetValueType(), eAmf::True);
|
|
ASSERT_EQ(array.Get<bool>("false")->GetValueType(), eAmf::False);
|
|
|
|
// Test associative can remove values
|
|
array.Remove("true");
|
|
ASSERT_EQ(array.GetAssociative().size(), 1);
|
|
ASSERT_EQ(array.Get<bool>("true"), nullptr);
|
|
ASSERT_EQ(array.Get<bool>("false")->GetValueType(), eAmf::False);
|
|
|
|
array.Remove("false");
|
|
ASSERT_EQ(array.GetAssociative().size(), 0);
|
|
ASSERT_EQ(array.Get<bool>("true"), nullptr);
|
|
ASSERT_EQ(array.Get<bool>("false"), nullptr);
|
|
|
|
// Test that multiple of the same key respect only the first element of that key
|
|
array.Insert("true", true);
|
|
array.Insert("true", false);
|
|
ASSERT_EQ(array.GetAssociative().size(), 1);
|
|
ASSERT_EQ(array.Get<bool>("true")->GetValueType(), eAmf::True);
|
|
array.Remove("true");
|
|
|
|
// Now test the dense portion
|
|
// Get some out of bounds values and cast to incorrect template types
|
|
array.Push(true);
|
|
array.Push(false);
|
|
|
|
ASSERT_EQ(array.GetDense().size(), 2);
|
|
ASSERT_EQ(array.Get<bool>(0)->GetValueType(), eAmf::True);
|
|
ASSERT_EQ(array.Get<std::string>(0), nullptr);
|
|
ASSERT_EQ(array.Get<bool>(1)->GetValueType(), eAmf::False);
|
|
ASSERT_EQ(array.Get<bool>(155), nullptr);
|
|
|
|
array.Pop();
|
|
|
|
ASSERT_EQ(array.GetDense().size(), 1);
|
|
ASSERT_EQ(array.Get<bool>(0)->GetValueType(), eAmf::True);
|
|
ASSERT_EQ(array.Get<std::string>(0), nullptr);
|
|
ASSERT_EQ(array.Get<bool>(1), nullptr);
|
|
|
|
array.Pop();
|
|
|
|
ASSERT_EQ(array.GetDense().size(), 0);
|
|
ASSERT_EQ(array.Get<bool>(0), nullptr);
|
|
ASSERT_EQ(array.Get<std::string>(0), nullptr);
|
|
ASSERT_EQ(array.Get<bool>(1), nullptr);
|
|
}
|
|
|
|
TEST(dCommonTests, AMF3InsertionAssociativeTest) {
|
|
AMFArrayValue array;
|
|
array.Insert("CString", "string");
|
|
array.Insert("String", std::string("string"));
|
|
array.Insert("False", false);
|
|
array.Insert("True", true);
|
|
array.Insert<int32_t>("Integer", 42U);
|
|
array.Insert("Double", 42.0);
|
|
array.InsertArray("Array");
|
|
array.Insert<std::vector<uint32_t>>("Undefined", {});
|
|
array.Insert("Null", nullptr);
|
|
|
|
ASSERT_EQ(array.Get<const char*>("CString")->GetValueType(), eAmf::String);
|
|
ASSERT_EQ(array.Get<std::string>("String")->GetValueType(), eAmf::String);
|
|
ASSERT_EQ(array.Get<bool>("False")->GetValueType(), eAmf::False);
|
|
ASSERT_EQ(array.Get<bool>("True")->GetValueType(), eAmf::True);
|
|
ASSERT_EQ(array.Get<int32_t>("Integer")->GetValueType(), eAmf::Integer);
|
|
ASSERT_EQ(array.Get<double>("Double")->GetValueType(), eAmf::Double);
|
|
ASSERT_EQ(array.GetArray("Array")->GetValueType(), eAmf::Array);
|
|
ASSERT_EQ(array.Get<std::nullptr_t>("Null")->GetValueType(), eAmf::Null);
|
|
ASSERT_EQ(array.Get<std::vector<uint32_t>>("Undefined")->GetValueType(), eAmf::Undefined);
|
|
}
|
|
|
|
TEST(dCommonTests, AMF3InsertionDenseTest) {
|
|
AMFArrayValue array;
|
|
array.Push<std::string>("string");
|
|
array.Push("CString");
|
|
array.Push(false);
|
|
array.Push(true);
|
|
array.Push<int32_t>(42U);
|
|
array.Push(42.0);
|
|
array.PushArray();
|
|
array.Push(nullptr);
|
|
array.Push<std::vector<uint32_t>>({});
|
|
|
|
ASSERT_EQ(array.Get<std::string>(0)->GetValueType(), eAmf::String);
|
|
ASSERT_EQ(array.Get<const char*>(1)->GetValueType(), eAmf::String);
|
|
ASSERT_EQ(array.Get<bool>(2)->GetValueType(), eAmf::False);
|
|
ASSERT_EQ(array.Get<bool>(3)->GetValueType(), eAmf::True);
|
|
ASSERT_EQ(array.Get<int32_t>(4)->GetValueType(), eAmf::Integer);
|
|
ASSERT_EQ(array.Get<double>(5)->GetValueType(), eAmf::Double);
|
|
ASSERT_EQ(array.GetArray(6)->GetValueType(), eAmf::Array);
|
|
ASSERT_EQ(array.Get<std::nullptr_t>(7)->GetValueType(), eAmf::Null);
|
|
ASSERT_EQ(array.Get<std::vector<uint32_t>>(8)->GetValueType(), eAmf::Undefined);
|
|
}
|