mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-19 17:11:32 +00:00
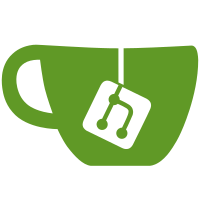
For Windows, the definition for a long is 32 bits, not 64 bits like on other operating systems. This caused an issue on Windows only where a number larger than 32 bits was attempted to be converted to a long, the WorldServer would crash. This commit replaces all instances of `stol` with `stoull` to further define a long and reduce ambiguity of number length.
49 lines
2.0 KiB
C++
49 lines
2.0 KiB
C++
#include "BaseFootRaceManager.h"
|
|
#include "EntityManager.h"
|
|
#include "Character.h"
|
|
|
|
void BaseFootRaceManager::OnStartup(Entity *self) {
|
|
// TODO: Add to FootRaceStarter group
|
|
}
|
|
|
|
void BaseFootRaceManager::OnFireEventServerSide(Entity *self, Entity *sender, std::string args, int32_t param1,
|
|
int32_t param2, int32_t param3) {
|
|
const auto splitArguments = GeneralUtils::SplitString(args, '_');
|
|
if (splitArguments.size() > 1) {
|
|
|
|
const auto eventName = splitArguments[0];
|
|
const auto player = EntityManager::Instance()->GetEntity(std::stoull(splitArguments[1]));
|
|
|
|
if (player != nullptr) {
|
|
if (eventName == "updatePlayer") {
|
|
UpdatePlayer(self, player->GetObjectID());
|
|
} else if (IsPlayerInActivity(self, player->GetObjectID())) {
|
|
if (eventName == "initialActivityScore") {
|
|
auto* character = player->GetCharacter();
|
|
if (character != nullptr) {
|
|
character->SetPlayerFlag(115, true);
|
|
}
|
|
|
|
SetActivityScore(self, player->GetObjectID(), 1);
|
|
} else if (eventName == "updatePlayerTrue") {
|
|
auto* character = player->GetCharacter();
|
|
if (character != nullptr) {
|
|
character->SetPlayerFlag(115, false);
|
|
}
|
|
|
|
UpdatePlayer(self, player->GetObjectID(), true);
|
|
} else if (eventName == "PlayerWon") {
|
|
auto* character = player->GetCharacter();
|
|
if (character != nullptr) {
|
|
character->SetPlayerFlag(115, false);
|
|
if (param2 != -1) // Certain footraces set a flag
|
|
character->SetPlayerFlag(param2, true);
|
|
}
|
|
|
|
StopActivity(self, player->GetObjectID(), 0, param1);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|