mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-20 01:21:32 +00:00
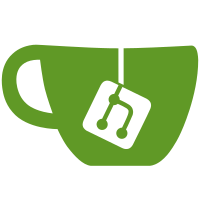
* CDClient cleanup and optimization - Use static function to get table name - Remove unused GetName function - Replace above function with a static GetTableName function - Remove verbose comments - Remove verbose initializers - Remove need to specify table name when getting a table by name - Remove unused typedef for mac and linux * Re-add unused table Convert tables to singletons - Convert all CDClient tables to singletons - Move Singleton.h to dCommon - Reduce header clutter in CDClientManager
47 lines
1.7 KiB
C++
47 lines
1.7 KiB
C++
#include "CDPhysicsComponentTable.h"
|
|
|
|
CDPhysicsComponentTable::CDPhysicsComponentTable(void) {
|
|
auto tableData = CDClientDatabase::ExecuteQuery("SELECT * FROM PhysicsComponent");
|
|
while (!tableData.eof()) {
|
|
CDPhysicsComponent* entry = new CDPhysicsComponent();
|
|
entry->id = tableData.getIntField("id", -1);
|
|
entry->bStatic = tableData.getIntField("static", -1) != 0;
|
|
entry->physicsAsset = tableData.getStringField("physics_asset", "");
|
|
UNUSED(entry->jump = tableData.getIntField("jump", -1) != 0);
|
|
UNUSED(entry->doublejump = tableData.getIntField("doublejump", -1) != 0);
|
|
entry->speed = tableData.getFloatField("speed", -1);
|
|
UNUSED(entry->rotSpeed = tableData.getFloatField("rotSpeed", -1));
|
|
entry->playerHeight = tableData.getFloatField("playerHeight");
|
|
entry->playerRadius = tableData.getFloatField("playerRadius");
|
|
entry->pcShapeType = tableData.getIntField("pcShapeType");
|
|
entry->collisionGroup = tableData.getIntField("collisionGroup");
|
|
UNUSED(entry->airSpeed = tableData.getFloatField("airSpeed"));
|
|
UNUSED(entry->boundaryAsset = tableData.getStringField("boundaryAsset"));
|
|
UNUSED(entry->jumpAirSpeed = tableData.getFloatField("jumpAirSpeed"));
|
|
UNUSED(entry->friction = tableData.getFloatField("friction"));
|
|
UNUSED(entry->gravityVolumeAsset = tableData.getStringField("gravityVolumeAsset"));
|
|
|
|
m_entries.insert(std::make_pair(entry->id, entry));
|
|
tableData.nextRow();
|
|
}
|
|
|
|
tableData.finalize();
|
|
}
|
|
|
|
CDPhysicsComponentTable::~CDPhysicsComponentTable() {
|
|
for (auto e : m_entries) {
|
|
if (e.second) delete e.second;
|
|
}
|
|
|
|
m_entries.clear();
|
|
}
|
|
|
|
CDPhysicsComponent* CDPhysicsComponentTable::GetByID(unsigned int componentID) {
|
|
for (auto e : m_entries) {
|
|
if (e.first == componentID) return e.second;
|
|
}
|
|
|
|
return nullptr;
|
|
}
|
|
|