mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-11-24 06:27:24 +00:00
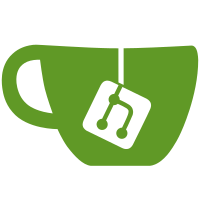
Utilizes `boost::interprocess` to share a map between worlds. The master server loads the table into memory, and the worlds hook into it when they want to get an entry. This solves the issue of skill delay, but introduces the third-party library Boost. There should be a conversation about the introduction of Boost — it is a large library and adds extra steps to the installation.
41 lines
987 B
C++
41 lines
987 B
C++
#pragma once
|
|
|
|
// Custom Classes
|
|
#include "CDTable.h"
|
|
#include <unordered_map>
|
|
#include <unordered_set>
|
|
|
|
/*!
|
|
\file CDBehaviorParameterTable.hpp
|
|
\brief Contains data for the BehaviorParameter table
|
|
*/
|
|
|
|
//! BehaviorParameter Entry Struct
|
|
struct CDBehaviorParameter {
|
|
int32_t behaviorID; //!< The Behavior ID
|
|
int32_t parameterID; //!< The Parameter ID
|
|
float value; //!< The value of the behavior template
|
|
};
|
|
|
|
//! BehaviorParameter table
|
|
class CDBehaviorParameterTable : public CDTable {
|
|
private:
|
|
std::unordered_map<size_t, float> m_Entries;
|
|
public:
|
|
static void CreateSharedMap();
|
|
|
|
//! Constructor
|
|
CDBehaviorParameterTable(void);
|
|
|
|
//! Destructor
|
|
~CDBehaviorParameterTable(void);
|
|
|
|
//! Returns the table's name
|
|
/*!
|
|
\return The table name
|
|
*/
|
|
std::string GetName(void) const override;
|
|
|
|
float GetEntry(const uint32_t behaviorID, const std::string& name, const float defaultValue = 0);
|
|
};
|