mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2025-07-07 04:00:02 +00:00
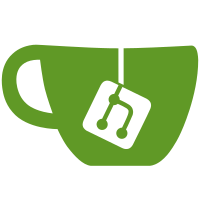
* Moved unrelated changes out of the TryParse PR branch * const correctness and cstdint type usage * removing a few "== nullptr" * amf constexpr, const-correctness, and attrib tagging * update to account for feedback * Fixing accidentally included header and hopefully fixing the MacOS issue too * try reordering the amf3 specializations to fix the MacOS issue again * Amf3 template class member func instantiation fix * try including only on macos * Using if constexpr rather than specialization * Trying a different solution for the instantiation problem * Remove #include "dPlatforms.h"
31 lines
1.1 KiB
C++
31 lines
1.1 KiB
C++
#include "BehaviorMessageBase.h"
|
|
|
|
#include "Amf3.h"
|
|
#include "BehaviorStates.h"
|
|
#include "dCommonVars.h"
|
|
|
|
BehaviorMessageBase::BehaviorMessageBase(AMFArrayValue* arguments) {
|
|
this->behaviorId = GetBehaviorIdFromArgument(arguments);
|
|
}
|
|
|
|
int32_t BehaviorMessageBase::GetBehaviorIdFromArgument(AMFArrayValue* const arguments) {
|
|
const char* const key = "BehaviorID";
|
|
const auto* const behaviorIDValue = arguments->Get<std::string>(key);
|
|
|
|
if (behaviorIDValue && behaviorIDValue->GetValueType() == eAmf::String) {
|
|
this->behaviorId =
|
|
GeneralUtils::TryParse<int32_t>(behaviorIDValue->GetValue()).value_or(this->behaviorId);
|
|
} else if (arguments->Get(key) && arguments->Get(key)->GetValueType() != eAmf::Undefined) {
|
|
throw std::invalid_argument("Unable to find behavior ID");
|
|
}
|
|
|
|
return this->behaviorId;
|
|
}
|
|
|
|
int32_t BehaviorMessageBase::GetActionIndexFromArgument(AMFArrayValue* const arguments, const std::string& keyName) const {
|
|
const auto* const actionIndexAmf = arguments->Get<double>(keyName);
|
|
if (!actionIndexAmf) throw std::invalid_argument("Unable to find actionIndex");
|
|
|
|
return static_cast<int32_t>(actionIndexAmf->GetValue());
|
|
}
|