mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-11-14 12:18:22 +00:00
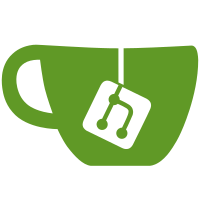
* Moved unrelated changes out of the TryParse PR branch * const correctness and cstdint type usage * removing a few "== nullptr" * amf constexpr, const-correctness, and attrib tagging * update to account for feedback * Fixing accidentally included header and hopefully fixing the MacOS issue too * try reordering the amf3 specializations to fix the MacOS issue again * Amf3 template class member func instantiation fix * try including only on macos * Using if constexpr rather than specialization * Trying a different solution for the instantiation problem * Remove #include "dPlatforms.h"
29 lines
1.2 KiB
C++
29 lines
1.2 KiB
C++
#include "AddStripMessage.h"
|
|
|
|
#include "Action.h"
|
|
|
|
AddStripMessage::AddStripMessage(AMFArrayValue* const arguments) : BehaviorMessageBase{ arguments } {
|
|
actionContext = ActionContext(arguments);
|
|
position = StripUiPosition(arguments);
|
|
|
|
auto* strip = arguments->GetArray("strip");
|
|
if (!strip) return;
|
|
|
|
auto* actions = strip->GetArray("actions");
|
|
if (!actions) return;
|
|
|
|
for (uint32_t actionNumber = 0; actionNumber < actions->GetDense().size(); actionNumber++) {
|
|
auto* actionValue = actions->GetArray(actionNumber);
|
|
if (!actionValue) continue;
|
|
|
|
actionsToAdd.push_back(Action(actionValue));
|
|
|
|
LOG_DEBUG("xPosition %f yPosition %f stripId %i stateId %i behaviorId %i t %s valueParameterName %s valueParameterString %s valueParameterDouble %f", position.GetX(), position.GetY(), actionContext.GetStripId(), actionContext.GetStateId(), behaviorId, actionsToAdd.back().GetType().c_str(), actionsToAdd.back().GetValueParameterName().c_str(), actionsToAdd.back().GetValueParameterString().c_str(), actionsToAdd.back().GetValueParameterDouble());
|
|
}
|
|
LOG_DEBUG("number of actions %i", actionsToAdd.size());
|
|
}
|
|
|
|
std::vector<Action> AddStripMessage::GetActionsToAdd() const {
|
|
return actionsToAdd;
|
|
}
|