mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2025-07-07 04:00:02 +00:00
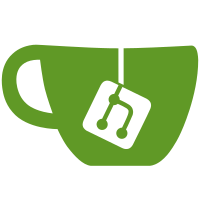
* CDClient cleanup and optimization - Use static function to get table name - Remove unused GetName function - Replace above function with a static GetTableName function - Remove verbose comments - Remove verbose initializers - Remove need to specify table name when getting a table by name - Remove unused typedef for mac and linux * Re-add unused table Convert tables to singletons - Convert all CDClient tables to singletons - Move Singleton.h to dCommon - Reduce header clutter in CDClientManager
36 lines
1.2 KiB
C++
36 lines
1.2 KiB
C++
#pragma once
|
|
|
|
// Custom Classes
|
|
#include "CDTable.h"
|
|
|
|
struct CDMissionTasks {
|
|
unsigned int id; //!< The Mission ID that the task belongs to
|
|
UNUSED(unsigned int locStatus); //!< ???
|
|
unsigned int taskType; //!< The task type
|
|
unsigned int target; //!< The mission target
|
|
std::string targetGroup; //!< The mission target group
|
|
int targetValue; //!< The target value
|
|
std::string taskParam1; //!< The task param 1
|
|
UNUSED(std::string largeTaskIcon); //!< ???
|
|
UNUSED(unsigned int IconID); //!< ???
|
|
unsigned int uid; //!< ???
|
|
UNUSED(unsigned int largeTaskIconID); //!< ???
|
|
UNUSED(bool localize); //!< Whether or not the task should be localized
|
|
UNUSED(std::string gate_version); //!< ???
|
|
};
|
|
|
|
class CDMissionTasksTable : public CDTable<CDMissionTasksTable> {
|
|
private:
|
|
std::vector<CDMissionTasks> entries;
|
|
|
|
public:
|
|
CDMissionTasksTable();
|
|
// Queries the table with a custom "where" clause
|
|
std::vector<CDMissionTasks> Query(std::function<bool(CDMissionTasks)> predicate);
|
|
|
|
std::vector<CDMissionTasks*> GetByMissionID(uint32_t missionID);
|
|
|
|
const std::vector<CDMissionTasks>& GetEntries(void) const;
|
|
};
|
|
|