mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-20 09:31:31 +00:00
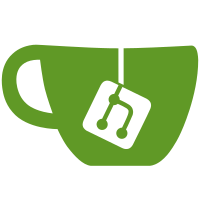
* CDClient cleanup and optimization - Use static function to get table name - Remove unused GetName function - Replace above function with a static GetTableName function - Remove verbose comments - Remove verbose initializers - Remove need to specify table name when getting a table by name - Remove unused typedef for mac and linux * Re-add unused table Convert tables to singletons - Convert all CDClient tables to singletons - Move Singleton.h to dCommon - Reduce header clutter in CDClientManager
41 lines
1.1 KiB
C++
41 lines
1.1 KiB
C++
#include "CDEmoteTable.h"
|
|
|
|
//! Constructor
|
|
CDEmoteTableTable::CDEmoteTableTable(void) {
|
|
auto tableData = CDClientDatabase::ExecuteQuery("SELECT * FROM Emotes");
|
|
while (!tableData.eof()) {
|
|
CDEmoteTable* entry = new CDEmoteTable();
|
|
entry->ID = tableData.getIntField("id", -1);
|
|
entry->animationName = tableData.getStringField("animationName", "");
|
|
entry->iconFilename = tableData.getStringField("iconFilename", "");
|
|
entry->channel = tableData.getIntField("channel", -1);
|
|
entry->locked = tableData.getIntField("locked", -1) != 0;
|
|
entry->localize = tableData.getIntField("localize", -1) != 0;
|
|
entry->locState = tableData.getIntField("locStatus", -1);
|
|
entry->gateVersion = tableData.getStringField("gate_version", "");
|
|
|
|
entries.insert(std::make_pair(entry->ID, entry));
|
|
tableData.nextRow();
|
|
}
|
|
|
|
tableData.finalize();
|
|
}
|
|
|
|
//! Destructor
|
|
CDEmoteTableTable::~CDEmoteTableTable(void) {
|
|
for (auto e : entries) {
|
|
if (e.second) delete e.second;
|
|
}
|
|
|
|
entries.clear();
|
|
}
|
|
|
|
CDEmoteTable* CDEmoteTableTable::GetEmote(int id) {
|
|
for (auto e : entries) {
|
|
if (e.first == id) return e.second;
|
|
}
|
|
|
|
return nullptr;
|
|
}
|
|
|