mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-11-10 02:08:20 +00:00
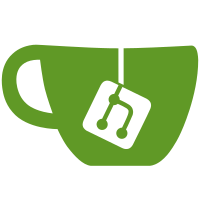
* moving branch * Add deleteinven slash command * Change name of BRICKS_IN_BBB * Use string_view instead of strcmp * Clean up include tree * Remove unneeded headers from PCH files Removes unneeded headers from pre-compiled headers. This increases compile time, however reduces development time for most files. * Update Entity.h * Update EntityManager.h * Update GameMessages.cpp * There it compiles now Co-authored-by: Aaron Kimbrell <aronwk.aaron@gmail.com>
95 lines
2.2 KiB
C++
95 lines
2.2 KiB
C++
#include "GfBanana.h"
|
|
|
|
#include "Entity.h"
|
|
#include "DestroyableComponent.h"
|
|
#include "EntityInfo.h"
|
|
#include "EntityManager.h"
|
|
|
|
void GfBanana::SpawnBanana(Entity* self) {
|
|
auto position = self->GetPosition();
|
|
const auto rotation = self->GetRotation();
|
|
|
|
position.y += 12;
|
|
position.x -= rotation.GetRightVector().x * 5;
|
|
position.z -= rotation.GetRightVector().z * 5;
|
|
|
|
EntityInfo info{};
|
|
|
|
info.pos = position;
|
|
info.rot = rotation;
|
|
info.lot = 6909;
|
|
info.spawnerID = self->GetObjectID();
|
|
|
|
auto* entity = EntityManager::Instance()->CreateEntity(info);
|
|
|
|
EntityManager::Instance()->ConstructEntity(entity);
|
|
|
|
self->SetVar(u"banana", entity->GetObjectID());
|
|
|
|
entity->AddDieCallback([self]() {
|
|
self->SetVar(u"banana", LWOOBJID_EMPTY);
|
|
|
|
self->AddTimer("bananaTimer", 30);
|
|
});
|
|
}
|
|
|
|
void GfBanana::OnStartup(Entity* self) {
|
|
SpawnBanana(self);
|
|
}
|
|
|
|
void GfBanana::OnHit(Entity* self, Entity* attacker) {
|
|
auto* destroyable = self->GetComponent<DestroyableComponent>();
|
|
|
|
destroyable->SetHealth(9999);
|
|
|
|
const auto bananaId = self->GetVar<LWOOBJID>(u"banana");
|
|
|
|
if (bananaId == LWOOBJID_EMPTY) return;
|
|
|
|
auto* bananaEntity = EntityManager::Instance()->GetEntity(bananaId);
|
|
|
|
if (bananaEntity == nullptr) {
|
|
self->SetVar(u"banana", LWOOBJID_EMPTY);
|
|
|
|
self->AddTimer("bananaTimer", 30);
|
|
|
|
return;
|
|
}
|
|
|
|
bananaEntity->SetPosition(bananaEntity->GetPosition() - NiPoint3::UNIT_Y * 8);
|
|
|
|
auto* bananaDestroyable = bananaEntity->GetComponent<DestroyableComponent>();
|
|
|
|
bananaDestroyable->SetHealth(0);
|
|
|
|
bananaDestroyable->Smash(attacker->GetObjectID());
|
|
|
|
/*
|
|
auto position = self->GetPosition();
|
|
const auto rotation = self->GetRotation();
|
|
|
|
position.y += 12;
|
|
position.x -= rotation.GetRightVector().x * 5;
|
|
position.z -= rotation.GetRightVector().z * 5;
|
|
|
|
EntityInfo info {};
|
|
|
|
info.pos = position;
|
|
info.rot = rotation;
|
|
info.lot = 6718;
|
|
info.spawnerID = self->GetObjectID();
|
|
|
|
auto* entity = EntityManager::Instance()->CreateEntity(info);
|
|
|
|
EntityManager::Instance()->ConstructEntity(entity, UNASSIGNED_SYSTEM_ADDRESS);
|
|
*/
|
|
|
|
EntityManager::Instance()->SerializeEntity(self);
|
|
}
|
|
|
|
void GfBanana::OnTimerDone(Entity* self, std::string timerName) {
|
|
if (timerName == "bananaTimer") {
|
|
SpawnBanana(self);
|
|
}
|
|
}
|