mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-19 17:11:32 +00:00
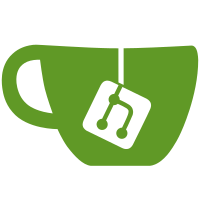
* Components: Make ComponentType inline Prevents the next commits ODR violation * Components: Add new components * Entity: Add headers inline script component ComponentType * Components: Flip constructor argument order Entity comes first always * Entity: Add generic AddComponent Allows for much easier adding of components and is error proof by not allowing the user to add more than 1 of a specific component type to an Entity. * Entity: Migrate all component constructors Move all to the new variadic templates AddComponent function to reduce clutter and ways the component map is modified. The new function makes no assumptions. Component is assumed to not exist and is checked for with operator[]. This will construct a null component which will then be newed if the component didnt exist, or it will just get the current component if it does already exist. No new component will be allocated or constructed if the component already exists and the already existing pointer is returned instead. * Entity: Add placement new For the case where the component may already exist, use a placement new to construct the component again, it would be constructed again, but would not need to go through the allocator. * Entity: Add comments on likely new code * Tests: Fix tests * Update Entity.cpp * Update SGCannon.cpp * Entity: call destructor when re-constructing * Update Entity.cpp Update Entity.cpp --------- Co-authored-by: Aaron Kimbrell <aronwk.aaron@gmail.com>
66 lines
1.5 KiB
C++
66 lines
1.5 KiB
C++
/*
|
|
* Darkflame Universe
|
|
* Copyright 2018
|
|
*/
|
|
|
|
#ifndef SCRIPTCOMPONENT_H
|
|
#define SCRIPTCOMPONENT_H
|
|
|
|
#include "CppScripts.h"
|
|
#include "Component.h"
|
|
#include <string>
|
|
#include "eReplicaComponentType.h"
|
|
|
|
class Entity;
|
|
|
|
/**
|
|
* Handles the loading and execution of server side scripts on entities, scripts were originally written in Lua,
|
|
* here they're written in C++
|
|
*/
|
|
class ScriptComponent : public Component {
|
|
public:
|
|
inline static const eReplicaComponentType ComponentType = eReplicaComponentType::SCRIPT;
|
|
|
|
ScriptComponent(Entity* parent, std::string scriptName, bool serialized, bool client = false);
|
|
~ScriptComponent() override;
|
|
|
|
void Serialize(RakNet::BitStream* outBitStream, bool bIsInitialUpdate) override;
|
|
|
|
/**
|
|
* Returns the script that's attached to this entity
|
|
* @return the script that's attached to this entity
|
|
*/
|
|
CppScripts::Script* GetScript();
|
|
|
|
/**
|
|
* Sets whether the entity should be serialized, unused
|
|
* @param var whether the entity should be serialized
|
|
*/
|
|
void SetSerialized(const bool var) { m_Serialized = var; }
|
|
|
|
/**
|
|
* Sets the script using a path by looking through dScripts for a script that matches
|
|
* @param scriptName the name of the script to find
|
|
*/
|
|
void SetScript(const std::string& scriptName);
|
|
|
|
private:
|
|
|
|
/**
|
|
* The script attached to this entity
|
|
*/
|
|
CppScripts::Script* m_Script;
|
|
|
|
/**
|
|
* Whether or not the comp should be serialized, unused
|
|
*/
|
|
bool m_Serialized;
|
|
|
|
/**
|
|
* Whether or not this script is a client script
|
|
*/
|
|
bool m_Client;
|
|
};
|
|
|
|
#endif // SCRIPTCOMPONENT_H
|