mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-19 09:01:31 +00:00
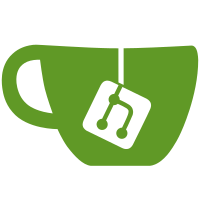
* fix serialization Was incorrect before. The only flags are if any data in the FrameStats has changed, you write them again. Velocities also do not use dirty flags for their values, they use a flag to determine if their velocity if zero or non-zero. if any velocity changes, re-write FrameStats. Tested that 2 players can see each other move as before, enemies move as before and players racing is identical as before. * Update HavokVehiclePhysicsComponent.cpp
81 lines
1.8 KiB
C++
81 lines
1.8 KiB
C++
#pragma once
|
|
|
|
#include "BitStream.h"
|
|
#include "Entity.h"
|
|
#include "PhysicsComponent.h"
|
|
#include "eReplicaComponentType.h"
|
|
#include "PositionUpdate.h"
|
|
|
|
/**
|
|
* Physics component for vehicles.
|
|
*/
|
|
class HavokVehiclePhysicsComponent : public PhysicsComponent {
|
|
public:
|
|
static constexpr eReplicaComponentType ComponentType = eReplicaComponentType::HAVOK_VEHICLE_PHYSICS;
|
|
|
|
HavokVehiclePhysicsComponent(Entity* parentEntity);
|
|
|
|
void Serialize(RakNet::BitStream& outBitStream, bool bIsInitialUpdate) override;
|
|
|
|
/**
|
|
* Sets the velocity
|
|
* @param vel the new velocity
|
|
*/
|
|
void SetVelocity(const NiPoint3& vel);
|
|
|
|
/**
|
|
* Gets the velocity
|
|
* @return the velocity
|
|
*/
|
|
const NiPoint3& GetVelocity() const { return m_Velocity; }
|
|
|
|
/**
|
|
* Sets the angular velocity
|
|
* @param vel the new angular velocity
|
|
*/
|
|
void SetAngularVelocity(const NiPoint3& vel);
|
|
|
|
/**
|
|
* Gets the angular velocity
|
|
* @return the angular velocity
|
|
*/
|
|
const NiPoint3& GetAngularVelocity() const { return m_AngularVelocity; }
|
|
|
|
/**
|
|
* Sets whether the vehicle is on the ground
|
|
* @param val whether the vehicle is on the ground
|
|
*/
|
|
void SetIsOnGround(bool val);
|
|
|
|
/**
|
|
* Gets whether the vehicle is on the ground
|
|
* @return whether the vehicle is on the ground
|
|
*/
|
|
const bool GetIsOnGround() const { return m_IsOnGround; }
|
|
|
|
/**
|
|
* Gets whether the vehicle is on rail
|
|
* @return whether the vehicle is on rail
|
|
*/
|
|
void SetIsOnRail(bool val);
|
|
|
|
/**
|
|
* Gets whether the vehicle is on rail
|
|
* @return whether the vehicle is on rail
|
|
*/
|
|
const bool GetIsOnRail() const { return m_IsOnRail; }
|
|
|
|
void SetRemoteInputInfo(const RemoteInputInfo&);
|
|
|
|
private:
|
|
NiPoint3 m_Velocity;
|
|
NiPoint3 m_AngularVelocity;
|
|
|
|
bool m_IsOnGround;
|
|
bool m_IsOnRail;
|
|
|
|
float m_SoftUpdate = 0;
|
|
uint32_t m_EndBehavior;
|
|
RemoteInputInfo m_RemoteInputInfo;
|
|
};
|