mirror of
https://github.com/DarkflameUniverse/DarkflameServer.git
synced 2024-09-19 09:01:31 +00:00
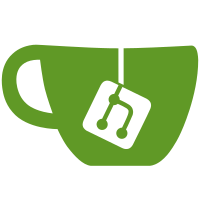
resolves a memory leak in BrickDatabase, adds stability to character save doc. Tested that saving manually via force-save, logout and /crash all saved my position and my removed banana as expected. The doc was always deleted on character destruction and on any updates, so this is just a semantic change (and now we no longer have new'd tinyxml2::documents on the heap)
54 lines
1.1 KiB
C++
54 lines
1.1 KiB
C++
#pragma once
|
|
|
|
#include "tinyxml2.h"
|
|
|
|
class Entity;
|
|
|
|
/**
|
|
* Component base class, provides methods for game loop updates, usage events and loading and saving to XML.
|
|
*/
|
|
class Component {
|
|
public:
|
|
Component(Entity* parent);
|
|
virtual ~Component();
|
|
|
|
/**
|
|
* Gets the owner of this component
|
|
* @return the owner of this component
|
|
*/
|
|
Entity* GetParent() const;
|
|
|
|
/**
|
|
* Updates the component in the game loop
|
|
* @param deltaTime time passed since last update
|
|
*/
|
|
virtual void Update(float deltaTime);
|
|
|
|
/**
|
|
* Event called when this component is being used, e.g. when some entity interacted with it
|
|
* @param originator
|
|
*/
|
|
virtual void OnUse(Entity* originator);
|
|
|
|
/**
|
|
* Save data from this componennt to character XML
|
|
* @param doc the document to write data to
|
|
*/
|
|
virtual void UpdateXml(tinyxml2::XMLDocument& doc);
|
|
|
|
/**
|
|
* Load base data for this component from character XML
|
|
* @param doc the document to read data from
|
|
*/
|
|
virtual void LoadFromXml(const tinyxml2::XMLDocument& doc);
|
|
|
|
virtual void Serialize(RakNet::BitStream& outBitStream, bool isConstruction);
|
|
|
|
protected:
|
|
|
|
/**
|
|
* The entity that owns this component
|
|
*/
|
|
Entity* m_Parent;
|
|
};
|