mirror of
https://github.com/yattee/yattee.git
synced 2024-11-10 08:18:19 +00:00
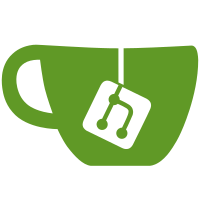
- player is now a separate window on macOS - add setting to disable pause when player is closed (fixes #40) - add PiP settings: * Close PiP when starting playing other video * Close PiP when player is opened * Close PiP and open player when application enters foreground (iOS/tvOS) (fixes #37) - new player placeholder when in PiP, context menu with exit option
80 lines
2.1 KiB
Swift
80 lines
2.1 KiB
Swift
import Foundation
|
|
import SwiftUI
|
|
|
|
struct MenuCommands: Commands {
|
|
@Binding var model: MenuModel
|
|
|
|
var body: some Commands {
|
|
navigationMenu
|
|
playbackMenu
|
|
}
|
|
|
|
private var navigationMenu: some Commands {
|
|
CommandGroup(before: .windowSize) {
|
|
Button("Favorites") {
|
|
model.navigation?.tabSelection = .favorites
|
|
}
|
|
.keyboardShortcut("1")
|
|
|
|
Button("Subscriptions") {
|
|
model.navigation?.tabSelection = .subscriptions
|
|
}
|
|
.disabled(subscriptionsDisabled)
|
|
.keyboardShortcut("2")
|
|
|
|
Button("Popular") {
|
|
model.navigation?.tabSelection = .popular
|
|
}
|
|
.disabled(!(model.accounts?.app.supportsPopular ?? true))
|
|
.keyboardShortcut("3")
|
|
|
|
Button("Trending") {
|
|
model.navigation?.tabSelection = .trending
|
|
}
|
|
.keyboardShortcut("4")
|
|
|
|
Button("Search") {
|
|
model.navigation?.tabSelection = .search
|
|
}
|
|
.keyboardShortcut("f")
|
|
|
|
Divider()
|
|
}
|
|
}
|
|
|
|
private var subscriptionsDisabled: Bool {
|
|
!(
|
|
(model.accounts?.app.supportsSubscriptions ?? false) && model.accounts?.signedIn ?? false
|
|
)
|
|
}
|
|
|
|
private var playbackMenu: some Commands {
|
|
CommandMenu("Playback") {
|
|
Button((model.player?.isPlaying ?? true) ? "Pause" : "Play") {
|
|
model.player?.togglePlay()
|
|
}
|
|
.disabled(model.player?.currentItem.isNil ?? true)
|
|
.keyboardShortcut("p")
|
|
|
|
Button("Play Next") {
|
|
model.player?.advanceToNextItem()
|
|
}
|
|
.disabled(model.player?.queue.isEmpty ?? true)
|
|
.keyboardShortcut("s")
|
|
|
|
Button(togglePlayerLabel) {
|
|
model.player?.togglePlayer()
|
|
}
|
|
.keyboardShortcut("o")
|
|
}
|
|
}
|
|
|
|
private var togglePlayerLabel: String {
|
|
#if os(macOS)
|
|
"Show Player"
|
|
#else
|
|
(model.player?.presentingPlayer ?? true) ? "Hide Player" : "Show Player"
|
|
#endif
|
|
}
|
|
}
|