mirror of
https://github.com/yattee/yattee.git
synced 2024-11-10 00:08:21 +00:00
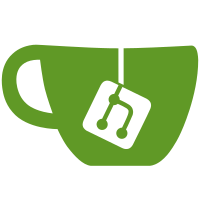
Chapters have their own section is the settings. The users can decide if chapter thumbnails should be shown or not. Also they can decide to only show thumbnails if they are not the same. The VideoDetailsView had to be modified, to avoid compiler type-checking errors.
85 lines
2.9 KiB
Swift
85 lines
2.9 KiB
Swift
import Foundation
|
|
import SDWebImageSwiftUI
|
|
import SwiftUI
|
|
|
|
struct ChaptersView: View {
|
|
@ObservedObject private var player = PlayerModel.shared
|
|
@Binding var expand: Bool
|
|
let chaptersHaveImages: Bool
|
|
let showThumbnails: Bool
|
|
|
|
var chapters: [Chapter] {
|
|
player.videoForDisplay?.chapters ?? []
|
|
}
|
|
|
|
var body: some View {
|
|
if !chapters.isEmpty {
|
|
if chaptersHaveImages, showThumbnails {
|
|
#if os(tvOS)
|
|
List {
|
|
Section {
|
|
ForEach(chapters) { chapter in
|
|
ChapterViewTVOS(chapter: chapter)
|
|
}
|
|
}
|
|
.listRowBackground(Color.clear)
|
|
}
|
|
.listStyle(.plain)
|
|
#else
|
|
ScrollView(.horizontal) {
|
|
LazyHStack(spacing: 20) { chapterViews(for: chapters[...]) }.padding(.horizontal, 15)
|
|
}
|
|
#endif
|
|
} else if expand {
|
|
#if os(tvOS)
|
|
Section {
|
|
ForEach(chapters) { chapter in
|
|
ChapterViewTVOS(chapter: chapter)
|
|
}
|
|
}
|
|
#else
|
|
Section { chapterViews(for: chapters[...]) }.padding(.horizontal)
|
|
#endif
|
|
} else {
|
|
#if os(iOS)
|
|
Button(action: {
|
|
self.expand.toggle()
|
|
}) {
|
|
Section {
|
|
chapterViews(for: chapters.prefix(3), opacity: 0.3, clickable: false)
|
|
}.padding(.horizontal)
|
|
}
|
|
#elseif os(macOS)
|
|
Section {
|
|
chapterViews(for: chapters.prefix(3), opacity: 0.3, clickable: false)
|
|
}.padding(.horizontal)
|
|
#else
|
|
Section {
|
|
ForEach(chapters) { chapter in
|
|
ChapterViewTVOS(chapter: chapter)
|
|
}
|
|
}
|
|
#endif
|
|
}
|
|
}
|
|
}
|
|
|
|
#if !os(tvOS)
|
|
private func chapterViews(for chaptersToShow: ArraySlice<Chapter>, opacity: Double = 1.0, clickable: Bool = true) -> some View {
|
|
ForEach(Array(chaptersToShow.indices), id: \.self) { index in
|
|
let chapter = chaptersToShow[index]
|
|
ChapterView(chapter: chapter, chapterIndex: index, showThumbnail: showThumbnails)
|
|
.opacity(index == 0 ? 1.0 : opacity)
|
|
.allowsHitTesting(clickable)
|
|
}
|
|
}
|
|
#endif
|
|
}
|
|
|
|
struct ChaptersView_Previews: PreviewProvider {
|
|
static var previews: some View {
|
|
ChaptersView(expand: .constant(false), chaptersHaveImages: false, showThumbnails: true)
|
|
.injectFixtureEnvironmentObjects()
|
|
}
|
|
}
|