mirror of
https://github.com/gtxaspec/wz_mini_hacks.git
synced 2024-09-19 16:01:31 +00:00
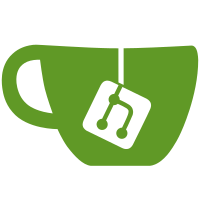
To reduce the chance that a bad firmware image is created and used, the following functionality has been implemented: 1. Check md5 sums of local files in addition to downloaded files 2. Copy a backup of the original firmware, instead of removing it 3. Do not make an image unless all parts are present 4. Only output success message if the image file exists The above fixes an issue where if a provided firmware zipfile is unable to be read, the script continues on, generates a bad demo.bin file, and suggests the file is good for loading into the camera. The above updates help prevent this from occurring
50 lines
1.5 KiB
Bash
Executable File
50 lines
1.5 KiB
Bash
Executable File
#!/bin/sh
|
|
|
|
#custom for V2
|
|
|
|
set -x
|
|
|
|
ACTION=$1
|
|
|
|
KERNEL_OFFSET=$((64))
|
|
ROOTFS_OFFSET=$((2097216))
|
|
DRIVER_OFFSET=$((5570624))
|
|
APPFS_OFFSET=$((6225984))
|
|
|
|
if [ "$ACTION" = "unpack" ]; then
|
|
DEMO_IN=$2
|
|
OUT_DIR=$3
|
|
|
|
dd if=${DEMO_IN} of=$OUT_DIR/kernel.bin skip=$KERNEL_OFFSET count=$(($ROOTFS_OFFSET-$KERNEL_OFFSET)) bs=1
|
|
md5sum $OUT_DIR/kernel.bin
|
|
|
|
dd if=${DEMO_IN} of=$OUT_DIR/rootfs.bin skip=$ROOTFS_OFFSET count=$(($DRIVER_OFFSET-$ROOTFS_OFFSET)) bs=1
|
|
md5sum $OUT_DIR/rootfs.bin
|
|
|
|
dd if=${DEMO_IN} of=$OUT_DIR/driver.bin skip=$DRIVER_OFFSET count=$(($APPFS_OFFSET-$DRIVER_OFFSET)) bs=1
|
|
md5sum $OUT_DIR/driver.bin
|
|
|
|
if [ "$(uname -s)" = "Darwin" ]; then
|
|
IMAGE_END=$(($(stat -f %z ${DEMO_IN})))
|
|
else
|
|
IMAGE_END=$(($(stat -c %s ${DEMO_IN})))
|
|
fi
|
|
|
|
dd if=${DEMO_IN} of=$OUT_DIR/appfs.bin skip=$APPFS_OFFSET count=$(($IMAGE_END-$APPFS_OFFSET)) bs=1
|
|
md5sum $OUT_DIR/appfs.bin
|
|
|
|
elif [ "$ACTION" = "pack" ]; then
|
|
TMP_DIR=$2
|
|
DEMO_OUT=$3
|
|
|
|
#need to pad kernel is its smaller than the stock kernel size, 2097152 bytes
|
|
dd if=/dev/zero of=$TMP_DIR/kernel.bin bs=1 count=1 seek=2097151
|
|
|
|
#only run mkimage if cat succeeds, otherwise it's possible that a bad image is created
|
|
cat $TMP_DIR/kernel.bin $TMP_DIR/rootfs.bin $TMP_DIR/driver.bin $TMP_DIR/appfs.bin > $TMP_DIR/flash.bin && \
|
|
mkimage -A MIPS -O linux -T firmware -C none -a 0 -e 0 -n jz_fw -d $TMP_DIR/flash.bin $DEMO_OUT
|
|
|
|
else
|
|
echo "Unknown action '$ACTION'"
|
|
fi
|