mirror of
https://github.com/python-kasa/python-kasa.git
synced 2025-07-07 04:00:05 +00:00
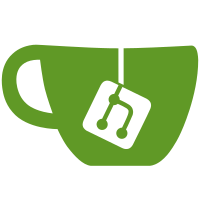
In order to support the ks240 which has children for the fan and light components, this PR adds those modules at the parent level and hides the children so it looks like a single device to consumers. It also decides which modules not to take from the child because the child does not support them even though it say it does. It does this for now via a fixed list, e.g. `Time`, `Firmware` etc. Also adds fixtures from two versions and corresponding tests.
64 lines
1.8 KiB
Python
64 lines
1.8 KiB
Python
"""Implementation of brightness module."""
|
|
|
|
from __future__ import annotations
|
|
|
|
from typing import TYPE_CHECKING
|
|
|
|
from ...feature import Feature
|
|
from ..smartmodule import SmartModule
|
|
|
|
if TYPE_CHECKING:
|
|
from ..smartdevice import SmartDevice
|
|
|
|
|
|
BRIGHTNESS_MIN = 1
|
|
BRIGHTNESS_MAX = 100
|
|
|
|
|
|
class Brightness(SmartModule):
|
|
"""Implementation of brightness module."""
|
|
|
|
REQUIRED_COMPONENT = "brightness"
|
|
|
|
def __init__(self, device: SmartDevice, module: str):
|
|
super().__init__(device, module)
|
|
self._add_feature(
|
|
Feature(
|
|
device,
|
|
"Brightness",
|
|
container=self,
|
|
attribute_getter="brightness",
|
|
attribute_setter="set_brightness",
|
|
minimum_value=BRIGHTNESS_MIN,
|
|
maximum_value=BRIGHTNESS_MAX,
|
|
type=Feature.Type.Number,
|
|
category=Feature.Category.Primary,
|
|
)
|
|
)
|
|
|
|
def query(self) -> dict:
|
|
"""Query to execute during the update cycle."""
|
|
# Brightness is contained in the main device info response.
|
|
return {}
|
|
|
|
@property
|
|
def brightness(self):
|
|
"""Return current brightness."""
|
|
return self.data["brightness"]
|
|
|
|
async def set_brightness(self, brightness: int):
|
|
"""Set the brightness."""
|
|
if not isinstance(brightness, int) or not (
|
|
BRIGHTNESS_MIN <= brightness <= BRIGHTNESS_MAX
|
|
):
|
|
raise ValueError(
|
|
f"Invalid brightness value: {brightness} "
|
|
f"(valid range: {BRIGHTNESS_MIN}-{BRIGHTNESS_MAX}%)"
|
|
)
|
|
|
|
return await self.call("set_device_info", {"brightness": brightness})
|
|
|
|
async def _check_supported(self):
|
|
"""Additional check to see if the module is supported by the device."""
|
|
return "brightness" in self.data
|