mirror of
https://github.com/python-kasa/python-kasa.git
synced 2024-12-23 11:43:34 +00:00
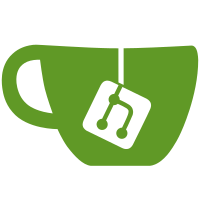
* Refactor devices into subpackages and deprecate old names * Tweak and add tests * Fix linting * Remove duplicate implementations affecting project coverage * Update post review * Add device base class attributes and rename subclasses * Rename Module to BaseModule * Remove has_emeter_history * Fix missing _time in init * Update post review * Fix test_readmeexamples * Fix erroneously duped files * Clean up iot and smart imports * Update post latest review * Tweak Device docstring
55 lines
1.4 KiB
Python
55 lines
1.4 KiB
Python
"""Provides the current time and timezone information."""
|
|
from datetime import datetime
|
|
|
|
from ...exceptions import SmartDeviceException
|
|
from .module import IotModule, merge
|
|
|
|
|
|
class Time(IotModule):
|
|
"""Implements the timezone settings."""
|
|
|
|
def query(self):
|
|
"""Request time and timezone."""
|
|
q = self.query_for_command("get_time")
|
|
|
|
merge(q, self.query_for_command("get_timezone"))
|
|
return q
|
|
|
|
@property
|
|
def time(self) -> datetime:
|
|
"""Return current device time."""
|
|
res = self.data["get_time"]
|
|
return datetime(
|
|
res["year"],
|
|
res["month"],
|
|
res["mday"],
|
|
res["hour"],
|
|
res["min"],
|
|
res["sec"],
|
|
)
|
|
|
|
@property
|
|
def timezone(self):
|
|
"""Return current timezone."""
|
|
res = self.data["get_timezone"]
|
|
return res
|
|
|
|
async def get_time(self):
|
|
"""Return current device time."""
|
|
try:
|
|
res = await self.call("get_time")
|
|
return datetime(
|
|
res["year"],
|
|
res["month"],
|
|
res["mday"],
|
|
res["hour"],
|
|
res["min"],
|
|
res["sec"],
|
|
)
|
|
except SmartDeviceException:
|
|
return None
|
|
|
|
async def get_timezone(self):
|
|
"""Request timezone information from the device."""
|
|
return await self.call("get_timezone")
|