mirror of
https://github.com/python-kasa/python-kasa.git
synced 2024-12-23 19:53:34 +00:00
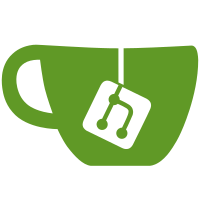
Addresses stability issues on older hw device versions - Handles module timeout errors better by querying modules individually on errors and disabling problematic modules like Firmware that go out to the internet to get updates. - Addresses an issue with the Led module on P100 hardware version 1.0 which appears to have a memory leak and will cause the device to crash after approximately 500 calls. - Delays updates of modules that do not have regular changes like LightPreset and LightEffect and enables them to be updated on the next update cycle only if required values have changed.
53 lines
1.5 KiB
Python
53 lines
1.5 KiB
Python
"""Module for led controls."""
|
|
|
|
from __future__ import annotations
|
|
|
|
from ...interfaces.led import Led as LedInterface
|
|
from ..smartmodule import SmartModule, allow_update_after
|
|
|
|
|
|
class Led(SmartModule, LedInterface):
|
|
"""Implementation of led controls."""
|
|
|
|
REQUIRED_COMPONENT = "led"
|
|
QUERY_GETTER_NAME = "get_led_info"
|
|
# Led queries can cause device to crash on P100
|
|
MINIMUM_UPDATE_INTERVAL_SECS = 60 * 60
|
|
|
|
def query(self) -> dict:
|
|
"""Query to execute during the update cycle."""
|
|
return {self.QUERY_GETTER_NAME: {"led_rule": None}}
|
|
|
|
@property
|
|
def mode(self):
|
|
"""LED mode setting.
|
|
|
|
"always", "never", "night_mode"
|
|
"""
|
|
return self.data["led_rule"]
|
|
|
|
@property
|
|
def led(self):
|
|
"""Return current led status."""
|
|
return self.data["led_rule"] != "never"
|
|
|
|
@allow_update_after
|
|
async def set_led(self, enable: bool):
|
|
"""Set led.
|
|
|
|
This should probably be a select with always/never/nightmode.
|
|
"""
|
|
rule = "always" if enable else "never"
|
|
return await self.call("set_led_info", dict(self.data, **{"led_rule": rule}))
|
|
|
|
@property
|
|
def night_mode_settings(self):
|
|
"""Night mode settings."""
|
|
return {
|
|
"start": self.data["start_time"],
|
|
"end": self.data["end_time"],
|
|
"type": self.data["night_mode_type"],
|
|
"sunrise_offset": self.data["sunrise_offset"],
|
|
"sunset_offset": self.data["sunset_offset"],
|
|
}
|