mirror of
https://github.com/python-kasa/python-kasa.git
synced 2024-12-23 11:43:34 +00:00
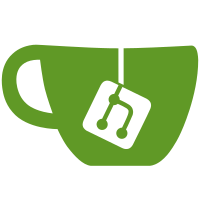
Devices not connected to the internet will either error when querying firmware queries (e.g. P300) or return misleading information (e.g. P100). This PR adds the cloud connect query to the initial queries and bypasses the firmware module if not connected.
41 lines
1.1 KiB
Python
41 lines
1.1 KiB
Python
"""Implementation of cloud module."""
|
|
|
|
from __future__ import annotations
|
|
|
|
from typing import TYPE_CHECKING
|
|
|
|
from ...exceptions import SmartErrorCode
|
|
from ...feature import Feature, FeatureType
|
|
from ..smartmodule import SmartModule
|
|
|
|
if TYPE_CHECKING:
|
|
from ..smartdevice import SmartDevice
|
|
|
|
|
|
class CloudModule(SmartModule):
|
|
"""Implementation of cloud module."""
|
|
|
|
QUERY_GETTER_NAME = "get_connect_cloud_state"
|
|
REQUIRED_COMPONENT = "cloud_connect"
|
|
|
|
def __init__(self, device: SmartDevice, module: str):
|
|
super().__init__(device, module)
|
|
|
|
self._add_feature(
|
|
Feature(
|
|
device,
|
|
"Cloud connection",
|
|
container=self,
|
|
attribute_getter="is_connected",
|
|
icon="mdi:cloud",
|
|
type=FeatureType.BinarySensor,
|
|
)
|
|
)
|
|
|
|
@property
|
|
def is_connected(self):
|
|
"""Return True if device is connected to the cloud."""
|
|
if isinstance(self.data, SmartErrorCode):
|
|
return False
|
|
return self.data["status"] == 0
|