mirror of
https://github.com/python-kasa/python-kasa.git
synced 2024-12-22 19:23:34 +00:00
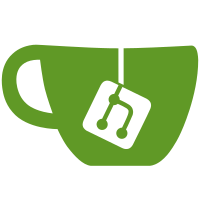
* Move tests to device-type specific test files to make improvements more approachable * protocol: remove the port parameter from query, as there are no other known ports, fix docstrings * Revise docstrings, remove superfluous information and remove unused methods ({set,get_icon} and set_time) * cli: indent device output to make it more easily readable when having multiple devices * remove adjust flake8 ignores (we have no setup.py anymore) * pyproject: include cli tool to coverage, add config for interrogate (docstring coverage) * bulb: raise exception on color_temp error cases instead of returning zero values * improve bulb tests, simplify conftest * strip: rename plugs property to children and move it to smartdevice
73 lines
2.2 KiB
Python
73 lines
2.2 KiB
Python
"""Module for dimmers (currently only HS220)."""
|
|
from typing import Any, Dict
|
|
|
|
from kasa.smartdevice import DeviceType, SmartDeviceException, requires_update
|
|
from kasa.smartplug import SmartPlug
|
|
|
|
|
|
class SmartDimmer(SmartPlug):
|
|
"""Representation of a TP-Link Smart Dimmer.
|
|
|
|
Dimmers work similarly to plugs, but provide also support for
|
|
adjusting the brightness. This class extends SmartPlug interface.
|
|
|
|
Example:
|
|
```
|
|
dimmer = SmartDimmer("192.168.1.105")
|
|
await dimmer.turn_on()
|
|
print("Current brightness: %s" % dimmer.brightness)
|
|
|
|
await dimmer.set_brightness(100)
|
|
```
|
|
|
|
Refer to SmartPlug for the full API.
|
|
"""
|
|
|
|
def __init__(self, host: str) -> None:
|
|
super().__init__(host)
|
|
self._device_type = DeviceType.Dimmer
|
|
|
|
@property # type: ignore
|
|
@requires_update
|
|
def brightness(self) -> int:
|
|
"""Return current brightness on dimmers.
|
|
|
|
Will return a range between 0 - 100.
|
|
"""
|
|
if not self.is_dimmable:
|
|
raise SmartDeviceException("Device is not dimmable.")
|
|
|
|
sys_info = self.sys_info
|
|
return int(sys_info["brightness"])
|
|
|
|
@requires_update
|
|
async def set_brightness(self, value: int):
|
|
"""Set the new dimmer brightness level in percentage."""
|
|
if not self.is_dimmable:
|
|
raise SmartDeviceException("Device is not dimmable.")
|
|
|
|
if not isinstance(value, int):
|
|
raise ValueError("Brightness must be integer, " "not of %s.", type(value))
|
|
elif 0 <= value <= 100:
|
|
return await self._query_helper(
|
|
"smartlife.iot.dimmer", "set_brightness", {"brightness": value}
|
|
)
|
|
else:
|
|
raise ValueError("Brightness value %s is not valid." % value)
|
|
|
|
@property # type: ignore
|
|
@requires_update
|
|
def is_dimmable(self) -> bool:
|
|
"""Whether the switch supports brightness changes."""
|
|
sys_info = self.sys_info
|
|
return "brightness" in sys_info
|
|
|
|
@property # type: ignore
|
|
@requires_update
|
|
def state_information(self) -> Dict[str, Any]:
|
|
"""Return switch-specific state information."""
|
|
info = super().state_information
|
|
info["Brightness"] = self.brightness
|
|
|
|
return info
|