mirror of
https://github.com/python-kasa/python-kasa.git
synced 2025-04-26 00:26:25 +00:00
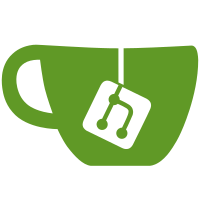
Some checks are pending
CI / Perform linting checks (3.13) (push) Waiting to run
CI / Python ${{ matrix.python-version}} on ${{ matrix.os }}${{ fromJSON('[" (extras)", ""]')[matrix.extras == ''] }} (false, macos-latest, 3.11) (push) Blocked by required conditions
CI / Python ${{ matrix.python-version}} on ${{ matrix.os }}${{ fromJSON('[" (extras)", ""]')[matrix.extras == ''] }} (false, macos-latest, 3.12) (push) Blocked by required conditions
CI / Python ${{ matrix.python-version}} on ${{ matrix.os }}${{ fromJSON('[" (extras)", ""]')[matrix.extras == ''] }} (false, macos-latest, 3.13) (push) Blocked by required conditions
CI / Python ${{ matrix.python-version}} on ${{ matrix.os }}${{ fromJSON('[" (extras)", ""]')[matrix.extras == ''] }} (false, ubuntu-latest, 3.11) (push) Blocked by required conditions
CI / Python ${{ matrix.python-version}} on ${{ matrix.os }}${{ fromJSON('[" (extras)", ""]')[matrix.extras == ''] }} (false, ubuntu-latest, 3.12) (push) Blocked by required conditions
CI / Python ${{ matrix.python-version}} on ${{ matrix.os }}${{ fromJSON('[" (extras)", ""]')[matrix.extras == ''] }} (false, ubuntu-latest, 3.13) (push) Blocked by required conditions
CI / Python ${{ matrix.python-version}} on ${{ matrix.os }}${{ fromJSON('[" (extras)", ""]')[matrix.extras == ''] }} (false, windows-latest, 3.11) (push) Blocked by required conditions
CI / Python ${{ matrix.python-version}} on ${{ matrix.os }}${{ fromJSON('[" (extras)", ""]')[matrix.extras == ''] }} (false, windows-latest, 3.12) (push) Blocked by required conditions
CI / Python ${{ matrix.python-version}} on ${{ matrix.os }}${{ fromJSON('[" (extras)", ""]')[matrix.extras == ''] }} (false, windows-latest, 3.13) (push) Blocked by required conditions
CI / Python ${{ matrix.python-version}} on ${{ matrix.os }}${{ fromJSON('[" (extras)", ""]')[matrix.extras == ''] }} (true, ubuntu-latest, 3.11) (push) Blocked by required conditions
CI / Python ${{ matrix.python-version}} on ${{ matrix.os }}${{ fromJSON('[" (extras)", ""]')[matrix.extras == ''] }} (true, ubuntu-latest, 3.12) (push) Blocked by required conditions
CI / Python ${{ matrix.python-version}} on ${{ matrix.os }}${{ fromJSON('[" (extras)", ""]')[matrix.extras == ''] }} (true, ubuntu-latest, 3.13) (push) Blocked by required conditions
CodeQL checks / Analyze (python) (push) Waiting to run
Add a common interface for the `alarm` module across `smart` and `smartcam` devices.
76 lines
1.9 KiB
Python
76 lines
1.9 KiB
Python
"""Module for base alarm module."""
|
|
|
|
from __future__ import annotations
|
|
|
|
from abc import ABC, abstractmethod
|
|
from typing import Annotated
|
|
|
|
from ..module import FeatureAttribute, Module
|
|
|
|
|
|
class Alarm(Module, ABC):
|
|
"""Base interface to represent an alarm module."""
|
|
|
|
@property
|
|
@abstractmethod
|
|
def alarm_sound(self) -> Annotated[str, FeatureAttribute()]:
|
|
"""Return current alarm sound."""
|
|
|
|
@abstractmethod
|
|
async def set_alarm_sound(self, sound: str) -> Annotated[dict, FeatureAttribute()]:
|
|
"""Set alarm sound.
|
|
|
|
See *alarm_sounds* for list of available sounds.
|
|
"""
|
|
|
|
@property
|
|
@abstractmethod
|
|
def alarm_sounds(self) -> list[str]:
|
|
"""Return list of available alarm sounds."""
|
|
|
|
@property
|
|
@abstractmethod
|
|
def alarm_volume(self) -> Annotated[int, FeatureAttribute()]:
|
|
"""Return alarm volume."""
|
|
|
|
@abstractmethod
|
|
async def set_alarm_volume(
|
|
self, volume: int
|
|
) -> Annotated[dict, FeatureAttribute()]:
|
|
"""Set alarm volume."""
|
|
|
|
@property
|
|
@abstractmethod
|
|
def alarm_duration(self) -> Annotated[int, FeatureAttribute()]:
|
|
"""Return alarm duration."""
|
|
|
|
@abstractmethod
|
|
async def set_alarm_duration(
|
|
self, duration: int
|
|
) -> Annotated[dict, FeatureAttribute()]:
|
|
"""Set alarm duration."""
|
|
|
|
@property
|
|
@abstractmethod
|
|
def active(self) -> bool:
|
|
"""Return true if alarm is active."""
|
|
|
|
@abstractmethod
|
|
async def play(
|
|
self,
|
|
*,
|
|
duration: int | None = None,
|
|
volume: int | None = None,
|
|
sound: str | None = None,
|
|
) -> dict:
|
|
"""Play alarm.
|
|
|
|
The optional *duration*, *volume*, and *sound* to override the device settings.
|
|
*duration* is in seconds.
|
|
See *alarm_sounds* for the list of sounds available for the device.
|
|
"""
|
|
|
|
@abstractmethod
|
|
async def stop(self) -> dict:
|
|
"""Stop alarm."""
|