mirror of
https://github.com/python-kasa/python-kasa.git
synced 2024-12-23 11:43:34 +00:00
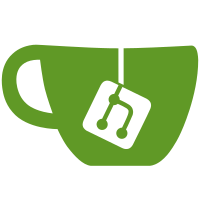
The initial steps to modularize the smartdevice. Modules are initialized based on the component negotiation, and each module can indicate which features it supports and which queries should be run during the update cycle.
55 lines
1.4 KiB
Python
55 lines
1.4 KiB
Python
"""Provides the current time and timezone information."""
|
|
from datetime import datetime
|
|
|
|
from ...exceptions import SmartDeviceException
|
|
from ..iotmodule import IotModule, merge
|
|
|
|
|
|
class Time(IotModule):
|
|
"""Implements the timezone settings."""
|
|
|
|
def query(self):
|
|
"""Request time and timezone."""
|
|
q = self.query_for_command("get_time")
|
|
|
|
merge(q, self.query_for_command("get_timezone"))
|
|
return q
|
|
|
|
@property
|
|
def time(self) -> datetime:
|
|
"""Return current device time."""
|
|
res = self.data["get_time"]
|
|
return datetime(
|
|
res["year"],
|
|
res["month"],
|
|
res["mday"],
|
|
res["hour"],
|
|
res["min"],
|
|
res["sec"],
|
|
)
|
|
|
|
@property
|
|
def timezone(self):
|
|
"""Return current timezone."""
|
|
res = self.data["get_timezone"]
|
|
return res
|
|
|
|
async def get_time(self):
|
|
"""Return current device time."""
|
|
try:
|
|
res = await self.call("get_time")
|
|
return datetime(
|
|
res["year"],
|
|
res["month"],
|
|
res["mday"],
|
|
res["hour"],
|
|
res["min"],
|
|
res["sec"],
|
|
)
|
|
except SmartDeviceException:
|
|
return None
|
|
|
|
async def get_timezone(self):
|
|
"""Request timezone information from the device."""
|
|
return await self.call("get_timezone")
|